Shape List - Person¶
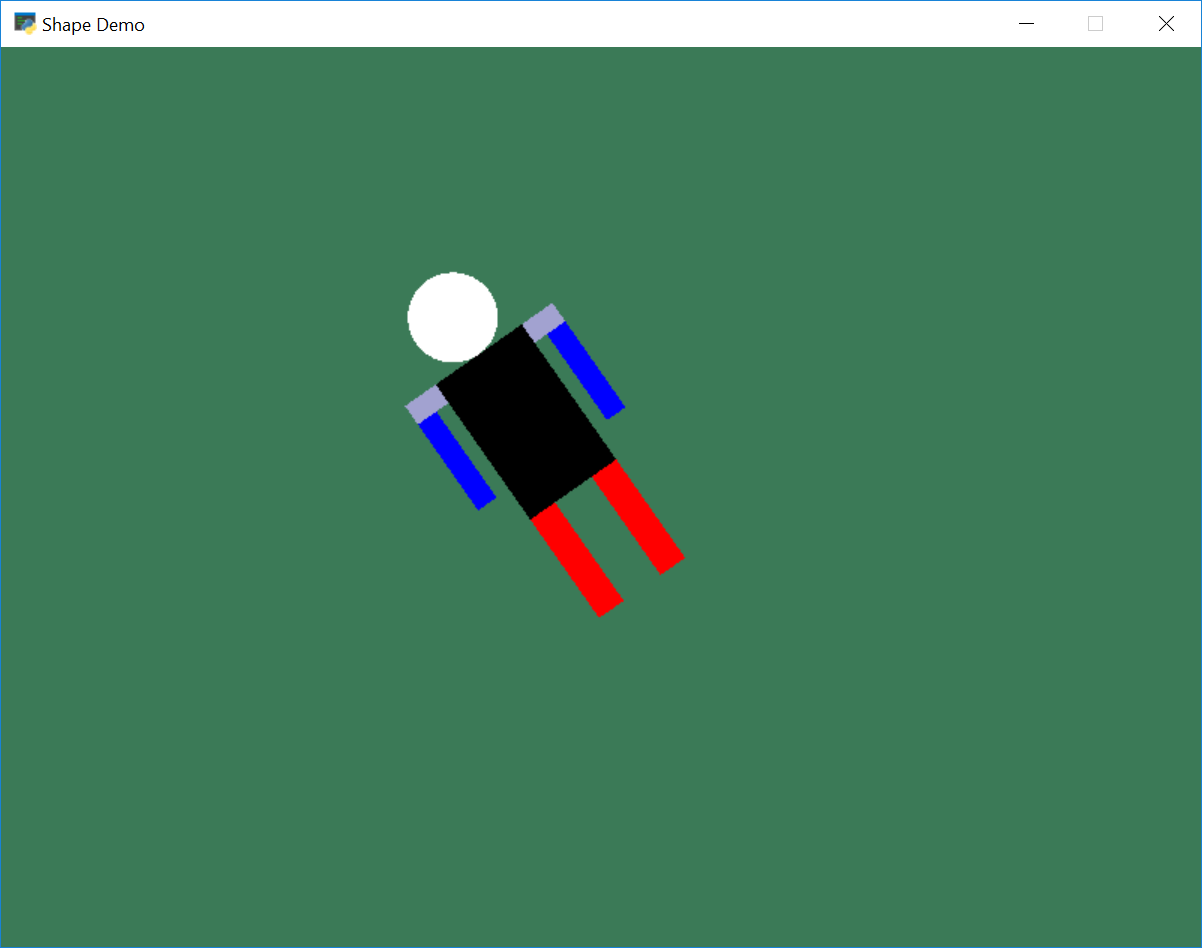
shape_list_demo_person.py¶
1"""
2Simple program showing how to use a shape list to create a more complex shape
3out of basic ones.
4
5If Python and Arcade are installed, this example can be run from the command line with:
6python -m arcade.examples.shape_list_demo_person
7"""
8import arcade
9
10SCREEN_WIDTH = 800
11SCREEN_HEIGHT = 600
12SCREEN_TITLE = "Shape List Demo Person"
13
14
15def make_person(head_radius,
16 chest_height,
17 chest_width,
18 leg_width,
19 leg_height,
20 arm_width,
21 arm_length,
22 arm_gap,
23 shoulder_height):
24
25 shape_list = arcade.ShapeElementList()
26
27 # Head
28 shape = arcade.create_ellipse_filled(0, chest_height / 2 + head_radius, head_radius, head_radius,
29 arcade.color.WHITE)
30 shape_list.append(shape)
31
32 # Chest
33 shape = arcade.create_rectangle_filled(0, 0, chest_width, chest_height, arcade.color.BLACK)
34 shape_list.append(shape)
35
36 # Left leg
37 shape = arcade.create_rectangle_filled(-(chest_width / 2) + leg_width / 2, -(chest_height / 2) - leg_height / 2,
38 leg_width, leg_height, arcade.color.RED)
39 shape_list.append(shape)
40
41 # Right leg
42 shape = arcade.create_rectangle_filled((chest_width / 2) - leg_width / 2, -(chest_height / 2) - leg_height / 2,
43 leg_width, leg_height, arcade.color.RED)
44 shape_list.append(shape)
45
46 # Left arm
47 shape = arcade.create_rectangle_filled(-(chest_width / 2) - arm_width / 2 - arm_gap,
48 (chest_height / 2) - arm_length / 2 - shoulder_height, arm_width, arm_length,
49 arcade.color.BLUE)
50 shape_list.append(shape)
51
52 # Left shoulder
53 shape = arcade.create_rectangle_filled(-(chest_width / 2) - (arm_gap + arm_width) / 2,
54 (chest_height / 2) - shoulder_height / 2, arm_gap + arm_width,
55 shoulder_height, arcade.color.BLUE_BELL)
56 shape_list.append(shape)
57
58 # Right arm
59 shape = arcade.create_rectangle_filled((chest_width / 2) + arm_width / 2 + arm_gap,
60 (chest_height / 2) - arm_length / 2 - shoulder_height, arm_width, arm_length,
61 arcade.color.BLUE)
62 shape_list.append(shape)
63
64 # Right shoulder
65 shape = arcade.create_rectangle_filled((chest_width / 2) + (arm_gap + arm_width) / 2,
66 (chest_height / 2) - shoulder_height / 2, arm_gap + arm_width,
67 shoulder_height, arcade.color.BLUE_BELL)
68 shape_list.append(shape)
69
70 return shape_list
71
72
73class MyGame(arcade.Window):
74 """ Main application class. """
75
76 def __init__(self):
77 """ Initializer """
78 # Call the parent class initializer
79 super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
80
81 head_radius = 30
82 chest_height = 110
83 chest_width = 70
84 leg_width = 20
85 leg_height = 80
86 arm_width = 15
87 arm_length = 70
88 arm_gap = 10
89 shoulder_height = 15
90
91 self.shape_list = make_person(head_radius,
92 chest_height,
93 chest_width,
94 leg_width,
95 leg_height,
96 arm_width,
97 arm_length,
98 arm_gap,
99 shoulder_height)
100
101 arcade.set_background_color(arcade.color.AMAZON)
102
103 def setup(self):
104
105 """ Set up the game and initialize the variables. """
106
107 def on_draw(self):
108 """
109 Render the screen.
110 """
111
112 # This command has to happen before we start drawing
113 arcade.start_render()
114
115 self.shape_list.draw()
116
117 def on_update(self, delta_time):
118 """ Movement and game logic """
119 self.shape_list.center_x += 1
120 self.shape_list.center_y += 1
121 self.shape_list.angle += .1
122
123
124def main():
125 window = MyGame()
126 window.setup()
127 arcade.run()
128
129
130if __name__ == "__main__":
131 main()