Pymunk Platformer
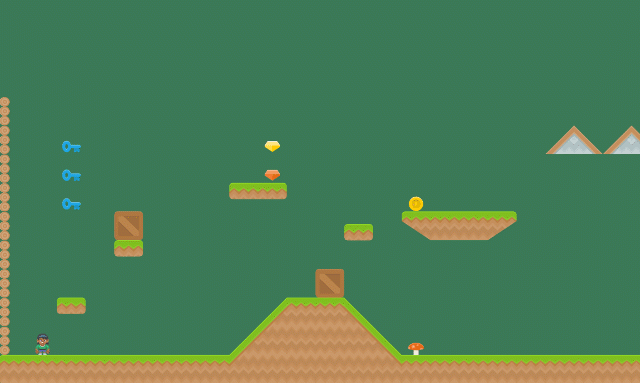
This tutorial covers how to write a platformer using Arcade and its Pymunk API. This tutorial assumes the you are somewhat familiar with Python, Arcade, and the Tiled Map Editor.
If you aren’t familiar with programming in Python, check out https://learn.arcade.academy
If you aren’t familiar with the Arcade library, work through the Simple Platformer.
If you aren’t familiar with the Tiled Map Editor, the Simple Platformer also introduces how to create a map with the Tiled Map Editor.
Common Issues
There are a few items with the Pymunk physics engine that should be pointed out before you get started:
Object overlap - A fast moving object is allowed to overlap with the object it collides with, and Pymunk will push them apart later. See collision bias for more information.
Pass-through - A fast moving object can pass through another object if its speed is so quick it never overlaps the other object between frames. See object tunneling.
When stepping the physics engine forward in time, the default is to move forward 1/60th of a second. Whatever increment is picked, increments should always be kept the same. Don’t use the variable delta_time from the
update
method as a unit, or results will be unstable and unpredictable. For a more accurate simulation, you can step forward 1/120th of a second twice per frame. This increases the time required, but takes more time to calculate.A sprite moving across a floor made up of many rectangles can get “caught” on the edges. The corner of the player sprite can get caught the corner of the floor sprite. To get around this, make sure the hit box for the bottom of the player sprite is rounded. Also, look into the possibility of merging horizontal rows of sprites.
Open a Window
To begin with, let’s start with a program that will use Arcade to open a blank window. It also has stubs for methods we’ll fill in later. Try this code and make sure you can run it. It should pop open a black window.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 | """
Example of Pymunk Physics Engine Platformer
"""
import arcade
SCREEN_TITLE = "PyMunk Platformer"
# Size of screen to show, in pixels
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
class GameWindow(arcade.Window):
""" Main Window """
def __init__(self, width, height, title):
""" Create the variables """
# Init the parent class
super().__init__(width, height, title)
def setup(self):
""" Set up everything with the game """
pass
def on_key_press(self, key, modifiers):
"""Called whenever a key is pressed. """
pass
def on_key_release(self, key, modifiers):
"""Called when the user releases a key. """
pass
def on_update(self, delta_time):
""" Movement and game logic """
pass
def on_draw(self):
""" Draw everything """
arcade.start_render()
def main():
""" Main function """
window = GameWindow(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
window.setup()
arcade.run()
if __name__ == "__main__":
main()
|
Create Constants
Now let’s set up the import
statements, and define the constants we are going
to use. In this case, we’ve got sprite tiles that are 128x128 pixels. They are
scaled down to 50% of the width and 50% of the height (scale of 0.5). The screen
size is set to 25x15 grid.
To keep things simple, this example will not scroll the screen with the player. See Simple Platformer or Move with a Scrolling Screen - Centered.
When you run this program, the screen should be larger.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | """
Example of Pymunk Physics Engine Platformer
"""
import math
from typing import Optional
import arcade
SCREEN_TITLE = "PyMunk Platformer"
# How big are our image tiles?
SPRITE_IMAGE_SIZE = 128
# Scale sprites up or down
SPRITE_SCALING_PLAYER = 0.5
SPRITE_SCALING_TILES = 0.5
# Scaled sprite size for tiles
SPRITE_SIZE = int(SPRITE_IMAGE_SIZE * SPRITE_SCALING_PLAYER)
# Size of grid to show on screen, in number of tiles
SCREEN_GRID_WIDTH = 25
SCREEN_GRID_HEIGHT = 15
# Size of screen to show, in pixels
SCREEN_WIDTH = SPRITE_SIZE * SCREEN_GRID_WIDTH
SCREEN_HEIGHT = SPRITE_SIZE * SCREEN_GRID_HEIGHT
class GameWindow(arcade.Window):
|
Create Instance Variables
Next, let’s create instance variables we are going to use, and set a background
color that’s green: arcade.color.AMAZON
If you aren’t familiar with type-casting on Python, you might not be familiar with lines of code like this:
self.player_list: Optional[arcade.SpriteList] = None
This means the player_list
attribute is going to be an instance of
SpriteList
or None
. If you don’t want to mess with typing, then
this code also works just as well:
self.player_list = None
Running this program should show the same window, but with a green background.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | class GameWindow(arcade.Window):
""" Main Window """
def __init__(self, width, height, title):
""" Create the variables """
# Init the parent class
super().__init__(width, height, title)
# Player sprite
self.player_sprite: Optional[arcade.Sprite] = None
# Sprite lists we need
self.player_list: Optional[arcade.SpriteList] = None
self.wall_list: Optional[arcade.SpriteList] = None
self.bullet_list: Optional[arcade.SpriteList] = None
self.item_list: Optional[arcade.SpriteList] = None
# Track the current state of what key is pressed
self.left_pressed: bool = False
self.right_pressed: bool = False
# Set background color
arcade.set_background_color(arcade.color.AMAZON)
|
Load and Display Map
To get started, create a map with the Tiled Map Editor. Place items that you don’t want to move, and to act as platforms in a layer named “Platforms”. Place items you want to push around in a layer called “Dynamic Items”. Name the file “pymunk_test_map.tmx” and place in the exact same directory as your code.
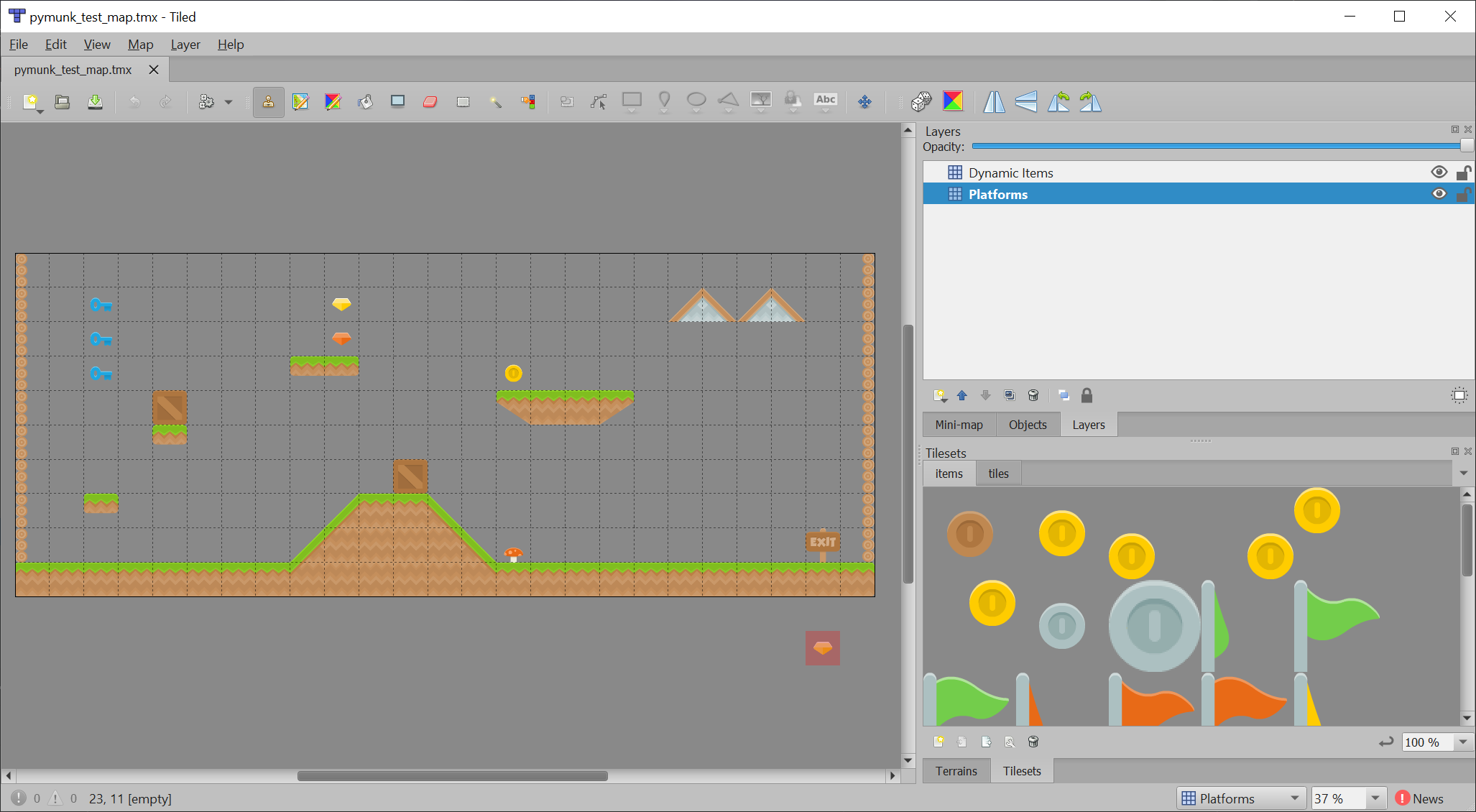
If you aren’t sure how to use the Tiled Map Editor, see Step 8 - Display The Score.
Now, in the setup
function, we are going add code to:
Create instances of
SpriteList
for each group of sprites we are doing to work with.Create the player sprite.
Read in the tiled map.
Make sprites from the layers in the tiled map.
Note
When making sprites from the tiled map layer, the name of the layer you load must match exactly with the layer created in the tiled map editor. It is case-sensitive.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | def setup(self):
""" Set up everything with the game """
# Create the sprite lists
self.player_list = arcade.SpriteList()
self.bullet_list = arcade.SpriteList()
# Map name
map_name = "pymunk_test_map.json"
# Load in TileMap
tile_map = arcade.load_tilemap(map_name, SPRITE_SCALING_TILES)
# Pull the sprite layers out of the tile map
self.wall_list = tile_map.sprite_lists["Platforms"]
self.item_list = tile_map.sprite_lists["Dynamic Items"]
# Create player sprite
self.player_sprite = arcade.Sprite(":resources:images/animated_characters/female_person/femalePerson_idle.png",
SPRITE_SCALING_PLAYER)
# Set player location
grid_x = 1
grid_y = 1
self.player_sprite.center_x = SPRITE_SIZE * grid_x + SPRITE_SIZE / 2
self.player_sprite.center_y = SPRITE_SIZE * grid_y + SPRITE_SIZE / 2
# Add to player sprite list
self.player_list.append(self.player_sprite)
|
There’s no point in having sprites if we don’t draw them, so in the on_draw
method, let’s draw out sprite lists.
1 2 3 4 5 6 7 | def on_draw(self):
""" Draw everything """
arcade.start_render()
self.wall_list.draw()
self.bullet_list.draw()
self.item_list.draw()
self.player_list.draw()
|
With the additions in the program below, running your program should show the tiled map you created:
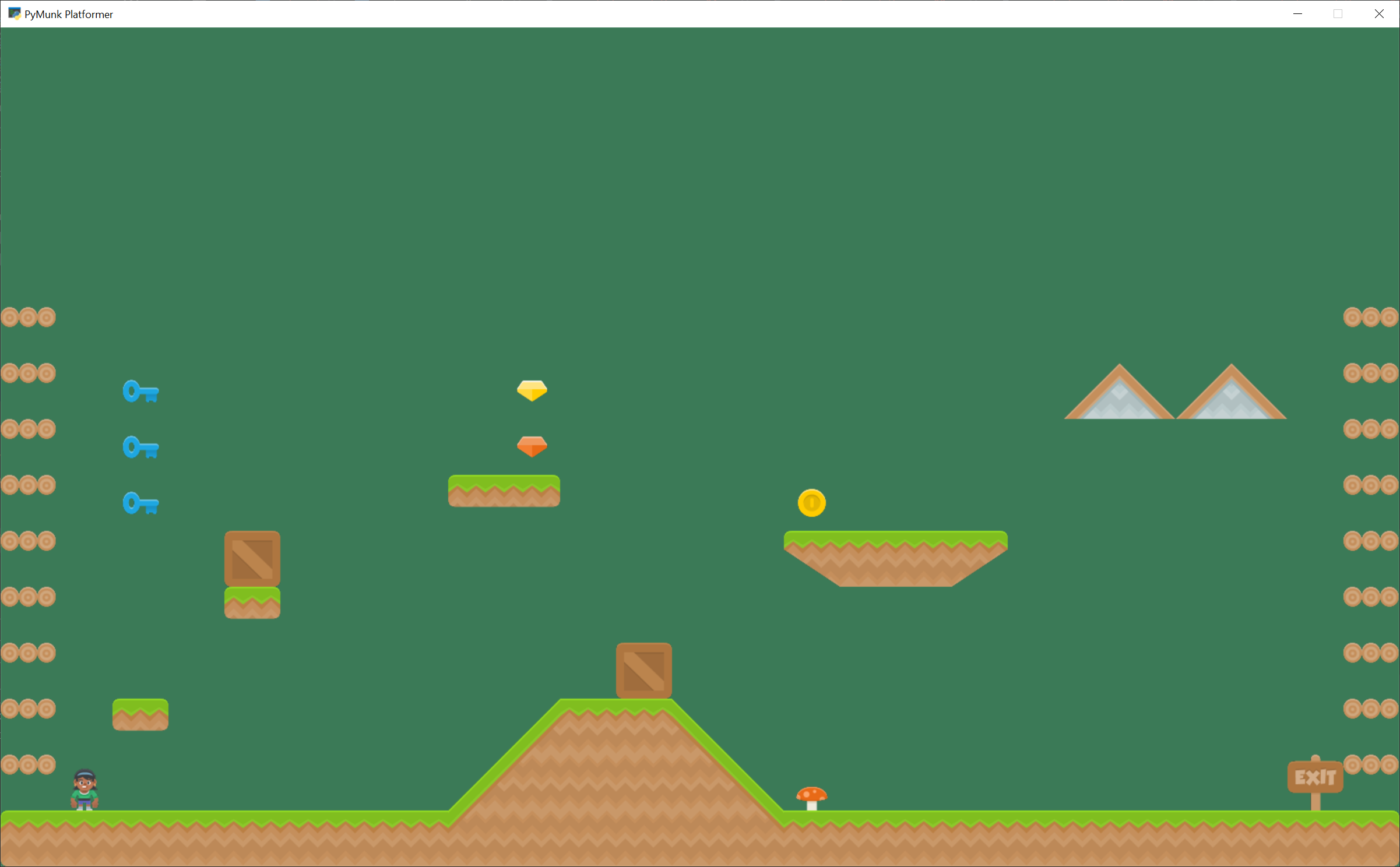
Add Physics Engine
The next step is to add in the physics engine.
First, add some constants for our physics. Here we are setting:
A constant for the force of gravity
Values for “damping”. A damping of 1.0 will cause an item to lose all it’s velocity once a force no longer applies to it. A damping of 0.5 causes 50% of speed to be lost in 1 second. A value of 0 is free-fall.
Values for friction. 0.0 is ice, 1.0 is like rubber.
Mass. Item default to 1. We make the player 2, so she can push items around easier.
Limits are the players horizontal and vertical speed. It is easier to play if the player is limited to a constant speed. And more realistic, because they aren’t on wheels.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | # --- Physics forces. Higher number, faster accelerating.
# Gravity
GRAVITY = 1500
# Damping - Amount of speed lost per second
DEFAULT_DAMPING = 1.0
PLAYER_DAMPING = 0.4
# Friction between objects
PLAYER_FRICTION = 1.0
WALL_FRICTION = 0.7
DYNAMIC_ITEM_FRICTION = 0.6
# Mass (defaults to 1)
PLAYER_MASS = 2.0
# Keep player from going too fast
PLAYER_MAX_HORIZONTAL_SPEED = 450
PLAYER_MAX_VERTICAL_SPEED = 1600
|
Second, add the following attributer in the __init__
method to hold our
physics engine:
1 2 |
# Physics engine
|
Third, in the setup
method we create the physics engine and add the sprites.
The player, walls, and dynamic items all have different properties so they are
added individually.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 | # Add to player sprite list
self.player_list.append(self.player_sprite)
# --- Pymunk Physics Engine Setup ---
# The default damping for every object controls the percent of velocity
# the object will keep each second. A value of 1.0 is no speed loss,
# 0.9 is 10% per second, 0.1 is 90% per second.
# For top-down games, this is basically the friction for moving objects.
# For platformers with gravity, this should probably be set to 1.0.
# Default value is 1.0 if not specified.
damping = DEFAULT_DAMPING
# Set the gravity. (0, 0) is good for outer space and top-down.
gravity = (0, -GRAVITY)
# Create the physics engine
self.physics_engine = arcade.PymunkPhysicsEngine(damping=damping,
gravity=gravity)
# Add the player.
# For the player, we set the damping to a lower value, which increases
# the damping rate. This prevents the character from traveling too far
# after the player lets off the movement keys.
# Setting the moment to PymunkPhysicsEngine.MOMENT_INF prevents it from
# rotating.
# Friction normally goes between 0 (no friction) and 1.0 (high friction)
# Friction is between two objects in contact. It is important to remember
# in top-down games that friction moving along the 'floor' is controlled
# by damping.
self.physics_engine.add_sprite(self.player_sprite,
friction=PLAYER_FRICTION,
mass=PLAYER_MASS,
moment=arcade.PymunkPhysicsEngine.MOMENT_INF,
collision_type="player",
max_horizontal_velocity=PLAYER_MAX_HORIZONTAL_SPEED,
max_vertical_velocity=PLAYER_MAX_VERTICAL_SPEED)
# Create the walls.
# By setting the body type to PymunkPhysicsEngine.STATIC the walls can't
# move.
# Movable objects that respond to forces are PymunkPhysicsEngine.DYNAMIC
# PymunkPhysicsEngine.KINEMATIC objects will move, but are assumed to be
# repositioned by code and don't respond to physics forces.
# Dynamic is default.
self.physics_engine.add_sprite_list(self.wall_list,
friction=WALL_FRICTION,
collision_type="wall",
body_type=arcade.PymunkPhysicsEngine.STATIC)
# Create the items
|
Fourth, in the on_update
method we call the physics engine’s step
method.
1 2 3 | """Called when the user releases a key. """
pass
|
If you run the program, and you have dynamic items that are up in the air, you should see them fall when the game starts.
Add Player Movement
Next step is to get the player moving. In this section we’ll cover how to move left and right. In the next section we’ll show how to jump.
The force that we will move the player is defined as PLAYER_MOVE_FORCE_ON_GROUND
.
We’ll apply a different force later, if the player happens to be airborne.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | # Force applied while on the ground
PLAYER_MOVE_FORCE_ON_GROUND = 8000
class GameWindow(arcade.Window):
""" Main Window """
def __init__(self, width, height, title):
""" Create the variables """
# Init the parent class
super().__init__(width, height, title)
# Player sprite
self.player_sprite: Optional[arcade.Sprite] = None
# Sprite lists we need
self.player_list: Optional[arcade.SpriteList] = None
self.wall_list: Optional[arcade.SpriteList] = None
self.bullet_list: Optional[arcade.SpriteList] = None
self.item_list: Optional[arcade.SpriteList] = None
# Track the current state of what key is pressed
self.left_pressed: bool = False
self.right_pressed: bool = False
|
We need to track if the left/right keys are held down. To do this we define
instance variables left_pressed
and right_pressed
. These are set to
appropriate values in the key press and release handlers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | collision_type="item")
def on_key_press(self, key, modifiers):
"""Called whenever a key is pressed. """
if key == arcade.key.LEFT:
self.left_pressed = True
elif key == arcade.key.RIGHT:
self.right_pressed = True
def on_key_release(self, key, modifiers):
"""Called when the user releases a key. """
if key == arcade.key.LEFT:
self.left_pressed = False
|
Finally, we need to apply the correct force in on_update
. Force is specified
in a tuple with horizontal force first, and vertical force second.
We also set the friction when we are moving to zero, and when we are not moving to 1. This is important to get realistic movement.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | self.right_pressed = False
def on_update(self, delta_time):
""" Movement and game logic """
# Update player forces based on keys pressed
if self.left_pressed and not self.right_pressed:
# Create a force to the left. Apply it.
force = (-PLAYER_MOVE_FORCE_ON_GROUND, 0)
self.physics_engine.apply_force(self.player_sprite, force)
# Set friction to zero for the player while moving
self.physics_engine.set_friction(self.player_sprite, 0)
elif self.right_pressed and not self.left_pressed:
# Create a force to the right. Apply it.
force = (PLAYER_MOVE_FORCE_ON_GROUND, 0)
self.physics_engine.apply_force(self.player_sprite, force)
# Set friction to zero for the player while moving
self.physics_engine.set_friction(self.player_sprite, 0)
else:
# Player's feet are not moving. Therefore up the friction so we stop.
self.physics_engine.set_friction(self.player_sprite, 1.0)
|
Add Player Jumping
To get the player to jump we need to:
Make sure the player is on the ground
Apply an impulse force to the player upward
Change the left/right force to the player while they are in the air.
We can see if a sprite has a sprite below it with the is_on_ground
function.
Otherwise we’ll be able to jump while we are in the air.
(Double-jumps would allow this once.)
If we don’t allow the player to move left-right while in the air, they player will be very hard to control. If we allow them to move left/right with the same force as on the ground, that’s typically too much. So we’ve got a different left/right force depending if we are in the air or not.
For the code changes, first we’ll define some constants:
1 2 3 4 5 | # Force applied when moving left/right in the air
PLAYER_MOVE_FORCE_IN_AIR = 900
# Strength of a jump
PLAYER_JUMP_IMPULSE = 1800
|
We’ll add logic that will apply the impulse force when we jump:
1 2 3 4 5 6 7 8 9 10 11 12 13 | friction=DYNAMIC_ITEM_FRICTION,
collision_type="item")
def on_key_press(self, key, modifiers):
"""Called whenever a key is pressed. """
if key == arcade.key.LEFT:
self.left_pressed = True
elif key == arcade.key.RIGHT:
self.right_pressed = True
elif key == arcade.key.UP:
# find out if player is standing on ground
if self.physics_engine.is_on_ground(self.player_sprite):
|
Then we will adjust the left/right force depending on if we are grounded or not:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | self.right_pressed = False
def on_update(self, delta_time):
""" Movement and game logic """
is_on_ground = self.physics_engine.is_on_ground(self.player_sprite)
# Update player forces based on keys pressed
if self.left_pressed and not self.right_pressed:
# Create a force to the left. Apply it.
if is_on_ground:
force = (-PLAYER_MOVE_FORCE_ON_GROUND, 0)
else:
force = (-PLAYER_MOVE_FORCE_IN_AIR, 0)
self.physics_engine.apply_force(self.player_sprite, force)
# Set friction to zero for the player while moving
self.physics_engine.set_friction(self.player_sprite, 0)
elif self.right_pressed and not self.left_pressed:
# Create a force to the right. Apply it.
if is_on_ground:
force = (PLAYER_MOVE_FORCE_ON_GROUND, 0)
else:
force = (PLAYER_MOVE_FORCE_IN_AIR, 0)
self.physics_engine.apply_force(self.player_sprite, force)
# Set friction to zero for the player while moving
self.physics_engine.set_friction(self.player_sprite, 0)
else:
# Player's feet are not moving. Therefore up the friction so we stop.
|
Add Player Animation
To create a player animation, we make a custom child class of Sprite
.
We load each frame of animation that we need, including a mirror image of it.
We will flip the player to face left or right. If the player is in the air, we’ll also change between a jump up and a falling graphics.
Because the physics engine works with small floating point numbers, it often flips above and below zero by small amounts. It is a good idea not to change the animation as the x and y float around zero. For that reason, in this code we have a “dead zone.” We don’t change the animation until it gets outside of that zone.
We also need to control how far the player moves before we change the walking animation, so that the feet appear in-sync with the ground.
1 2 3 4 5 6 7 8 9 | # Close enough to not-moving to have the animation go to idle.
DEAD_ZONE = 0.1
# Constants used to track if the player is facing left or right
RIGHT_FACING = 0
LEFT_FACING = 1
# How many pixels to move before we change the texture in the walking animation
DISTANCE_TO_CHANGE_TEXTURE = 20
|
Next, we create a Player
class that is a child to arcade.Sprite
. This
class will update the player animation.
The __init__
method loads all of the textures. Here we use Kenney.nl’s
Toon Characters 1 pack.
It has six different characters you can choose from with the same layout, so
it makes changing as simple as changing which line is enabled. There are
eight textures for walking, and textures for idle, jumping, and falling.
As the character can face left or right, we use arcade.load_texture_pair
which will load both a regular image, and one that’s mirrored.
For the multi-frame walking animation, we use an “odometer.” We need to move a certain number of pixels before changing the animation. If this value is too small our character moves her legs like Fred Flintstone, too large and it looks like you are ice skating. We keep track of the index of our current texture, 0-7 since there are eight of them.
Any sprite moved by the Pymunk engine will have its pymunk_moved
method
called. This can be used to update the animation.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 | class PlayerSprite(arcade.Sprite):
""" Player Sprite """
def __init__(self):
""" Init """
# Let parent initialize
super().__init__()
# Set our scale
self.scale = SPRITE_SCALING_PLAYER
# Images from Kenney.nl's Character pack
# main_path = ":resources:images/animated_characters/female_adventurer/femaleAdventurer"
main_path = ":resources:images/animated_characters/female_person/femalePerson"
# main_path = ":resources:images/animated_characters/male_person/malePerson"
# main_path = ":resources:images/animated_characters/male_adventurer/maleAdventurer"
# main_path = ":resources:images/animated_characters/zombie/zombie"
# main_path = ":resources:images/animated_characters/robot/robot"
# Load textures for idle standing
self.idle_texture_pair = arcade.load_texture_pair(f"{main_path}_idle.png")
self.jump_texture_pair = arcade.load_texture_pair(f"{main_path}_jump.png")
self.fall_texture_pair = arcade.load_texture_pair(f"{main_path}_fall.png")
# Load textures for walking
self.walk_textures = []
for i in range(8):
texture = arcade.load_texture_pair(f"{main_path}_walk{i}.png")
self.walk_textures.append(texture)
# Set the initial texture
self.texture = self.idle_texture_pair[0]
# Hit box will be set based on the first image used.
self.hit_box = self.texture.hit_box_points
# Default to face-right
self.character_face_direction = RIGHT_FACING
# Index of our current texture
self.cur_texture = 0
# How far have we traveled horizontally since changing the texture
self.x_odometer = 0
def pymunk_moved(self, physics_engine, dx, dy, d_angle):
""" Handle being moved by the pymunk engine """
# Figure out if we need to face left or right
if dx < -DEAD_ZONE and self.character_face_direction == RIGHT_FACING:
self.character_face_direction = LEFT_FACING
elif dx > DEAD_ZONE and self.character_face_direction == LEFT_FACING:
self.character_face_direction = RIGHT_FACING
# Are we on the ground?
is_on_ground = physics_engine.is_on_ground(self)
# Add to the odometer how far we've moved
self.x_odometer += dx
# Jumping animation
if not is_on_ground:
if dy > DEAD_ZONE:
self.texture = self.jump_texture_pair[self.character_face_direction]
return
elif dy < -DEAD_ZONE:
self.texture = self.fall_texture_pair[self.character_face_direction]
return
# Idle animation
if abs(dx) <= DEAD_ZONE:
self.texture = self.idle_texture_pair[self.character_face_direction]
return
# Have we moved far enough to change the texture?
if abs(self.x_odometer) > DISTANCE_TO_CHANGE_TEXTURE:
# Reset the odometer
self.x_odometer = 0
# Advance the walking animation
self.cur_texture += 1
if self.cur_texture > 7:
self.cur_texture = 0
self.texture = self.walk_textures[self.cur_texture][self.character_face_direction]
|
Important! At this point, we are still creating an instance of arcade.Sprite
and not PlayerSprite
. We need to go back to the setup
method and
replace the line that creates the player
instance with:
# Pull the sprite layers out of the tile map
self.wall_list = tile_map.sprite_lists["Platforms"]
A really common mistake I’ve seen programmers make (and made myself) is to forget that last part. Then you can spend a lot of time looking at the player class when the error is in the setup.
We also need to go back and change the data type for the player sprite attribute
in our __init__
method:
super().__init__(width, height, title)
Shoot Bullets
Getting the player to shoot something can add a lot to our game. To begin with we’ll define a few constants to use. How much force to shoot the bullet with, the bullet’s mass, and the gravity to use for the bullet.
If we use the same gravity for the bullet as everything else, it tends to drop too fast. We could set this to zero if we wanted it to not drop at all.
1 2 3 4 5 6 7 8 | # How much force to put on the bullet
BULLET_MOVE_FORCE = 4500
# Mass of the bullet
BULLET_MASS = 0.1
# Make bullet less affected by gravity
BULLET_GRAVITY = 300
|
Next, we’ll put in a mouse press handler to put in the bullet shooting code.
We need to:
Create the bullet sprite
We need to calculate the angle from the player to the mouse click
Create the bullet away from the player in the proper direction, as spawning it inside the player will confuse the physics engine
Add the bullet to the physics engine
Apply the force to the bullet to make if move. Note that as we angled the bullet we don’t need to angle the force.
Warning
Does your platformer scroll?
If your window scrolls, you need to add in the coordinate off-set or else the angle calculation will be incorrect.
Warning
Bullets don’t disappear yet!
If the bullet flies off-screen, it doesn’t go away and the physics engine still has to track it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 | def on_mouse_press(self, x, y, button, modifiers):
""" Called whenever the mouse button is clicked. """
bullet = arcade.SpriteSolidColor(20, 5, arcade.color.DARK_YELLOW)
self.bullet_list.append(bullet)
# Position the bullet at the player's current location
start_x = self.player_sprite.center_x
start_y = self.player_sprite.center_y
bullet.position = self.player_sprite.position
# Get from the mouse the destination location for the bullet
# IMPORTANT! If you have a scrolling screen, you will also need
# to add in self.view_bottom and self.view_left.
dest_x = x
dest_y = y
# Do math to calculate how to get the bullet to the destination.
# Calculation the angle in radians between the start points
# and end points. This is the angle the bullet will travel.
x_diff = dest_x - start_x
y_diff = dest_y - start_y
angle = math.atan2(y_diff, x_diff)
# What is the 1/2 size of this sprite, so we can figure out how far
# away to spawn the bullet
size = max(self.player_sprite.width, self.player_sprite.height) / 2
# Use angle to to spawn bullet away from player in proper direction
bullet.center_x += size * math.cos(angle)
bullet.center_y += size * math.sin(angle)
# Set angle of bullet
bullet.angle = math.degrees(angle)
# Gravity to use for the bullet
# If we don't use custom gravity, bullet drops too fast, or we have
# to make it go too fast.
# Force is in relation to bullet's angle.
bullet_gravity = (0, -BULLET_GRAVITY)
# Add the sprite. This needs to be done AFTER setting the fields above.
self.physics_engine.add_sprite(bullet,
mass=BULLET_MASS,
damping=1.0,
friction=0.6,
collision_type="bullet",
gravity=bullet_gravity,
elasticity=0.9)
# Add force to bullet
force = (BULLET_MOVE_FORCE, 0)
self.physics_engine.apply_force(bullet, force)
|
Destroy Bullets and Items
This section has two goals:
Get rid of the bullet if it flies off-screen
Handle collisions of the bullet and other items
Destroy Bullet If It Goes Off-Screen
First, we’ll create a custom bullet class. This class will define the
pymunk_moved
method, and check our location each time the bullet moves.
If our y value is too low, we’ll remove the bullet.
1 2 3 4 5 6 7 | class BulletSprite(arcade.SpriteSolidColor):
""" Bullet Sprite """
def pymunk_moved(self, physics_engine, dx, dy, d_angle):
""" Handle when the sprite is moved by the physics engine. """
# If the bullet falls below the screen, remove it
if self.center_y < -100:
self.remove_from_sprite_lists()
|
And, of course, once we create the bullet we have to update our code to use
it instead of the plain arcade.Sprite
class.
1 2 3 4 5 | if key == arcade.key.LEFT:
self.left_pressed = False
elif key == arcade.key.RIGHT:
self.right_pressed = False
|
Handle Collisions
To handle collisions, we can add custom collision handler call-backs. If you’ll remember when we added items to the physics engine, we gave each item a collision type, such as “wall” or “bullet” or “item”. We can write a function and register it to handle all bullet/wall collisions.
In this case, bullets that hit a wall go away. Bullets that hit items cause both the item and the bullet to go away. We could also add code to track damage to a sprite, only removing it after so much damage was applied. Even changing the texture depending on its health.
1 2 3 4 5 6 7 8 9 10 11 12 |
# Create the physics engine
self.physics_engine = arcade.PymunkPhysicsEngine(damping=damping,
gravity=gravity)
def wall_hit_handler(bullet_sprite, _wall_sprite, _arbiter, _space, _data):
""" Called for bullet/wall collision """
bullet_sprite.remove_from_sprite_lists()
self.physics_engine.add_collision_handler("bullet", "wall", post_handler=wall_hit_handler)
def item_hit_handler(bullet_sprite, item_sprite, _arbiter, _space, _data):
|
Add Moving Platforms
We can add support for moving platforms. Platforms can be added in an object layer. An object layer allows platforms to be placed anywhere, and not just on exact grid locations. Object layers also allow us to add custom properties for each tile we place.

Adding an object layer.
Once we have the tile placed, we can add custom properties for it. Click the ‘+’ icon and add properties for all or some of:
change_x
change_y
left_boundary
right_boundary
top_boundary
bottom_boundary
If these are named exact matches, they’ll automatically copy their values into the sprite attributes of the same name.
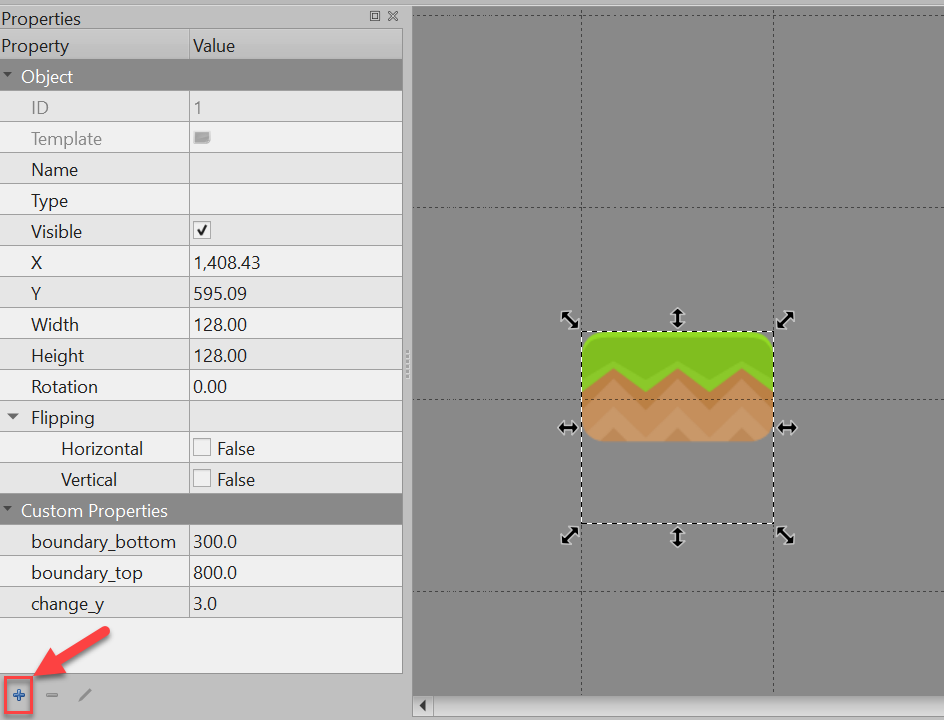
Adding custom properties.
Now we need to update our code. In GameWindow.__init__
add a line to create
an attribute for moving_sprites_list
:
self.wall_list: Optional[arcade.SpriteList] = None
In the setup
method, load in the sprite list from the tmx layer.
self.player_sprite.center_y = SPRITE_SIZE * grid_y + SPRITE_SIZE / 2
# Add to player sprite list
self.player_list.append(self.player_sprite)
Also in the setup
method, we need to add these sprites to the physics engine.
In this case we’ll add the sprites as KINEMATIC
. Static sprites don’t move.
Dynamic sprites move, and can have forces applied to them by other objects.
Kinematic sprites do move, but aren’t affected by other objects.
# Create the items
self.physics_engine.add_sprite_list(self.item_list,
friction=DYNAMIC_ITEM_FRICTION,
We need to draw the moving platform sprites. After adding this line, you should be able to run the program and see the sprites from this layer, even if they don’t move yet.
1 2 3 4 5 6 7 8 | def on_draw(self):
""" Draw everything """
arcade.start_render()
self.wall_list.draw()
self.moving_sprites_list.draw()
self.bullet_list.draw()
self.item_list.draw()
self.player_list.draw()
|
Next up, we need to get the sprites moving. First, we’ll check to see if there are any boundaries set, and if we need to reverse our direction.
After that we’ll create a velocity vector. Velocity is in pixels per second. In this case, I’m assuming the user set the velocity in pixels per frame in Tiled instead, so we’ll convert.
Warning
Changing center_x and center_y will not move the sprite. If you want to change
a sprite’s position, use the physics engine’s set_position
method.
Also, setting an item’s position “teleports” it there. The physics engine will happily move the object right into another object. Setting the item’s velocity instead will cause the physics engine to move the item, pushing any dynamic items out of the way.
self.physics_engine.set_friction(self.player_sprite, 1.0)
# Move items in the physics engine
self.physics_engine.step()
# For each moving sprite, see if we've reached a boundary and need to
# reverse course.
for moving_sprite in self.moving_sprites_list:
if moving_sprite.boundary_right and \
moving_sprite.change_x > 0 and \
moving_sprite.right > moving_sprite.boundary_right:
moving_sprite.change_x *= -1
elif moving_sprite.boundary_left and \
moving_sprite.change_x < 0 and \
moving_sprite.left > moving_sprite.boundary_left:
moving_sprite.change_x *= -1
if moving_sprite.boundary_top and \
moving_sprite.change_y > 0 and \
moving_sprite.top > moving_sprite.boundary_top:
moving_sprite.change_y *= -1
elif moving_sprite.boundary_bottom and \
moving_sprite.change_y < 0 and \
moving_sprite.bottom < moving_sprite.boundary_bottom:
moving_sprite.change_y *= -1
Add Ladders
The first step to adding ladders to our platformer is modify the __init__
to track some more items:
Have a reference to a list of ladder sprites
Add textures for a climbing animation
Keep track of our movement in the y direction
Add a boolean to track if we are on/off a ladder
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 | def __init__(self,
ladder_list: arcade.SpriteList,
hit_box_algorithm):
""" Init """
# Let parent initialize
super().__init__()
# Set our scale
self.scale = SPRITE_SCALING_PLAYER
# Images from Kenney.nl's Character pack
# main_path = ":resources:images/animated_characters/female_adventurer/femaleAdventurer"
main_path = ":resources:images/animated_characters/female_person/femalePerson"
# main_path = ":resources:images/animated_characters/male_person/malePerson"
# main_path = ":resources:images/animated_characters/male_adventurer/maleAdventurer"
# main_path = ":resources:images/animated_characters/zombie/zombie"
# main_path = ":resources:images/animated_characters/robot/robot"
# Load textures for idle standing
self.idle_texture_pair = arcade.load_texture_pair(f"{main_path}_idle.png",
hit_box_algorithm=hit_box_algorithm)
self.jump_texture_pair = arcade.load_texture_pair(f"{main_path}_jump.png")
self.fall_texture_pair = arcade.load_texture_pair(f"{main_path}_fall.png")
# Load textures for walking
self.walk_textures = []
for i in range(8):
texture = arcade.load_texture_pair(f"{main_path}_walk{i}.png")
self.walk_textures.append(texture)
# Load textures for climbing
self.climbing_textures = []
texture = arcade.load_texture(f"{main_path}_climb0.png")
self.climbing_textures.append(texture)
texture = arcade.load_texture(f"{main_path}_climb1.png")
self.climbing_textures.append(texture)
# Set the initial texture
self.texture = self.idle_texture_pair[0]
# Hit box will be set based on the first image used.
self.hit_box = self.texture.hit_box_points
# Default to face-right
self.character_face_direction = RIGHT_FACING
# Index of our current texture
self.cur_texture = 0
# How far have we traveled horizontally since changing the texture
self.x_odometer = 0
self.y_odometer = 0
self.ladder_list = ladder_list
self.is_on_ladder = False
|
Next, in our pymunk_moved
method we need to change physics when we are
on a ladder, and to update our player texture.
When we are on a ladder, we’ll turn off gravity, turn up damping, and turn down our max vertical velocity. If we are off the ladder, reset those attributes.
When we are on a ladder, but not on the ground, we’ll alternate between a couple climbing textures.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 | def pymunk_moved(self, physics_engine, dx, dy, d_angle):
""" Handle being moved by the pymunk engine """
# Figure out if we need to face left or right
if dx < -DEAD_ZONE and self.character_face_direction == RIGHT_FACING:
self.character_face_direction = LEFT_FACING
elif dx > DEAD_ZONE and self.character_face_direction == LEFT_FACING:
self.character_face_direction = RIGHT_FACING
# Are we on the ground?
is_on_ground = physics_engine.is_on_ground(self)
# Are we on a ladder?
if len(arcade.check_for_collision_with_list(self, self.ladder_list)) > 0:
if not self.is_on_ladder:
self.is_on_ladder = True
self.pymunk.gravity = (0, 0)
self.pymunk.damping = 0.0001
self.pymunk.max_vertical_velocity = PLAYER_MAX_HORIZONTAL_SPEED
else:
if self.is_on_ladder:
self.pymunk.damping = 1.0
self.pymunk.max_vertical_velocity = PLAYER_MAX_VERTICAL_SPEED
self.is_on_ladder = False
self.pymunk.gravity = None
# Add to the odometer how far we've moved
self.x_odometer += dx
self.y_odometer += dy
if self.is_on_ladder and not is_on_ground:
# Have we moved far enough to change the texture?
if abs(self.y_odometer) > DISTANCE_TO_CHANGE_TEXTURE:
# Reset the odometer
self.y_odometer = 0
# Advance the walking animation
self.cur_texture += 1
if self.cur_texture > 1:
self.cur_texture = 0
self.texture = self.climbing_textures[self.cur_texture]
return
# Jumping animation
if not is_on_ground:
if dy > DEAD_ZONE:
self.texture = self.jump_texture_pair[self.character_face_direction]
return
elif dy < -DEAD_ZONE:
self.texture = self.fall_texture_pair[self.character_face_direction]
return
# Idle animation
if abs(dx) <= DEAD_ZONE:
self.texture = self.idle_texture_pair[self.character_face_direction]
return
# Have we moved far enough to change the texture?
if abs(self.x_odometer) > DISTANCE_TO_CHANGE_TEXTURE:
# Reset the odometer
self.x_odometer = 0
# Advance the walking animation
self.cur_texture += 1
if self.cur_texture > 7:
self.cur_texture = 0
self.texture = self.walk_textures[self.cur_texture][self.character_face_direction]
|
Then we just need to add a few variables to the __init__
to track ladders:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | def __init__(self, width, height, title):
""" Create the variables """
# Init the parent class
super().__init__(width, height, title)
# Player sprite
self.player_sprite: Optional[PlayerSprite] = None
# Sprite lists we need
self.player_list: Optional[arcade.SpriteList] = None
self.wall_list: Optional[arcade.SpriteList] = None
self.bullet_list: Optional[arcade.SpriteList] = None
self.item_list: Optional[arcade.SpriteList] = None
self.moving_sprites_list: Optional[arcade.SpriteList] = None
self.ladder_list: Optional[arcade.SpriteList] = None
# Track the current state of what key is pressed
self.left_pressed: bool = False
self.right_pressed: bool = False
self.up_pressed: bool = False
self.down_pressed: bool = False
# Physics engine
self.physics_engine: Optional[arcade.PymunkPhysicsEngine] = None
# Set background color
arcade.set_background_color(arcade.color.AMAZON)
|
Then load the ladder layer in setup
:
tile_map = arcade.load_tilemap(map_name, SPRITE_SCALING_TILES)
# Pull the sprite layers out of the tile map
self.wall_list = tile_map.sprite_lists["Platforms"]
Also, pass the ladder list to the player class:
self.ladder_list = tile_map.sprite_lists["Ladders"]
self.moving_sprites_list = tile_map.sprite_lists['Moving Platforms']
Then change the jump button so that we don’t jump if we are on a ladder. Also, we want to track if the up key, or down key are pressed.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | def on_key_press(self, key, modifiers):
"""Called whenever a key is pressed. """
if key == arcade.key.LEFT:
self.left_pressed = True
elif key == arcade.key.RIGHT:
self.right_pressed = True
elif key == arcade.key.UP:
self.up_pressed = True
# find out if player is standing on ground, and not on a ladder
if self.physics_engine.is_on_ground(self.player_sprite) \
and not self.player_sprite.is_on_ladder:
# She is! Go ahead and jump
impulse = (0, PLAYER_JUMP_IMPULSE)
self.physics_engine.apply_impulse(self.player_sprite, impulse)
elif key == arcade.key.DOWN:
self.down_pressed = True
|
Add to the key up handler tracking for which key is pressed.
1 2 3 4 5 6 7 8 9 10 11 | def on_key_release(self, key, modifiers):
"""Called when the user releases a key. """
if key == arcade.key.LEFT:
self.left_pressed = False
elif key == arcade.key.RIGHT:
self.right_pressed = False
elif key == arcade.key.UP:
self.up_pressed = False
elif key == arcade.key.DOWN:
self.down_pressed = False
|
Next, change our update with new updates for the ladder.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
is_on_ground = self.physics_engine.is_on_ground(self.player_sprite)
# Update player forces based on keys pressed
if self.left_pressed and not self.right_pressed:
# Create a force to the left. Apply it.
if is_on_ground or self.player_sprite.is_on_ladder:
force = (-PLAYER_MOVE_FORCE_ON_GROUND, 0)
else:
force = (-PLAYER_MOVE_FORCE_IN_AIR, 0)
self.physics_engine.apply_force(self.player_sprite, force)
# Set friction to zero for the player while moving
self.physics_engine.set_friction(self.player_sprite, 0)
elif self.right_pressed and not self.left_pressed:
# Create a force to the right. Apply it.
if is_on_ground or self.player_sprite.is_on_ladder:
force = (PLAYER_MOVE_FORCE_ON_GROUND, 0)
else:
force = (PLAYER_MOVE_FORCE_IN_AIR, 0)
self.physics_engine.apply_force(self.player_sprite, force)
# Set friction to zero for the player while moving
self.physics_engine.set_friction(self.player_sprite, 0)
elif self.up_pressed and not self.down_pressed:
# Create a force to the right. Apply it.
if self.player_sprite.is_on_ladder:
force = (0, PLAYER_MOVE_FORCE_ON_GROUND)
self.physics_engine.apply_force(self.player_sprite, force)
# Set friction to zero for the player while moving
self.physics_engine.set_friction(self.player_sprite, 0)
elif self.down_pressed and not self.up_pressed:
# Create a force to the right. Apply it.
if self.player_sprite.is_on_ladder:
force = (0, -PLAYER_MOVE_FORCE_ON_GROUND)
self.physics_engine.apply_force(self.player_sprite, force)
# Set friction to zero for the player while moving
self.physics_engine.set_friction(self.player_sprite, 0)
else:
|
And, of course, don’t forget to draw the ladders:
1 2 3 4 5 6 7 8 9 | def on_draw(self):
""" Draw everything """
arcade.start_render()
self.wall_list.draw()
self.ladder_list.draw()
self.moving_sprites_list.draw()
self.bullet_list.draw()
self.item_list.draw()
self.player_list.draw()
|