Shader Toy Tutorial

Graphics cards can run programs written in the C-like language GLSL. These programs can be easily parallelized and run across the processors of the graphics card GPU.
Shaders take a bit of set-up to write. The ShaderToy website has standardized some of these and made it easier to experiment with writing shaders. The website is at:
Arcade includes additional code making it easier to run these ShaderToy shaders in an Arcade program. This short ShaderToy demo loads a GLSL file and displays it:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | import arcade
from arcade.experimental import Shadertoy
# Do the math to figure out our screen dimensions
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
SCREEN_TITLE = "ShaderToy Demo"
class MyGame(arcade.Window):
def __init__(self, width, height, title):
super().__init__(width, height, title, resizable=True)
self.time = 0
# file_name = "fractal_pyramid.glsl"
# file_name = "cyber_fuji_2020.glsl"
# file_name = "flame.glsl"
file_name = "star_nest.glsl"
file = open(file_name)
shader_sourcecode = file.read()
size = width, height
self.shadertoy = Shadertoy(size, shader_sourcecode)
self.mouse_pos = 0, 0
def on_draw(self):
arcade.start_render()
self.shadertoy.render(time=self.time, mouse_position=self.mouse_pos)
def on_update(self, dt):
# Keep track of elapsed time
self.time += dt
def on_mouse_drag(self, x, y, dx, dy, buttons, modifiers):
self.mouse_pos = x, y
if __name__ == "__main__":
MyGame(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
arcade.run()
|
You can click on the caption below the example shaders here to see the source code for the shader.
Some other sample shaders:
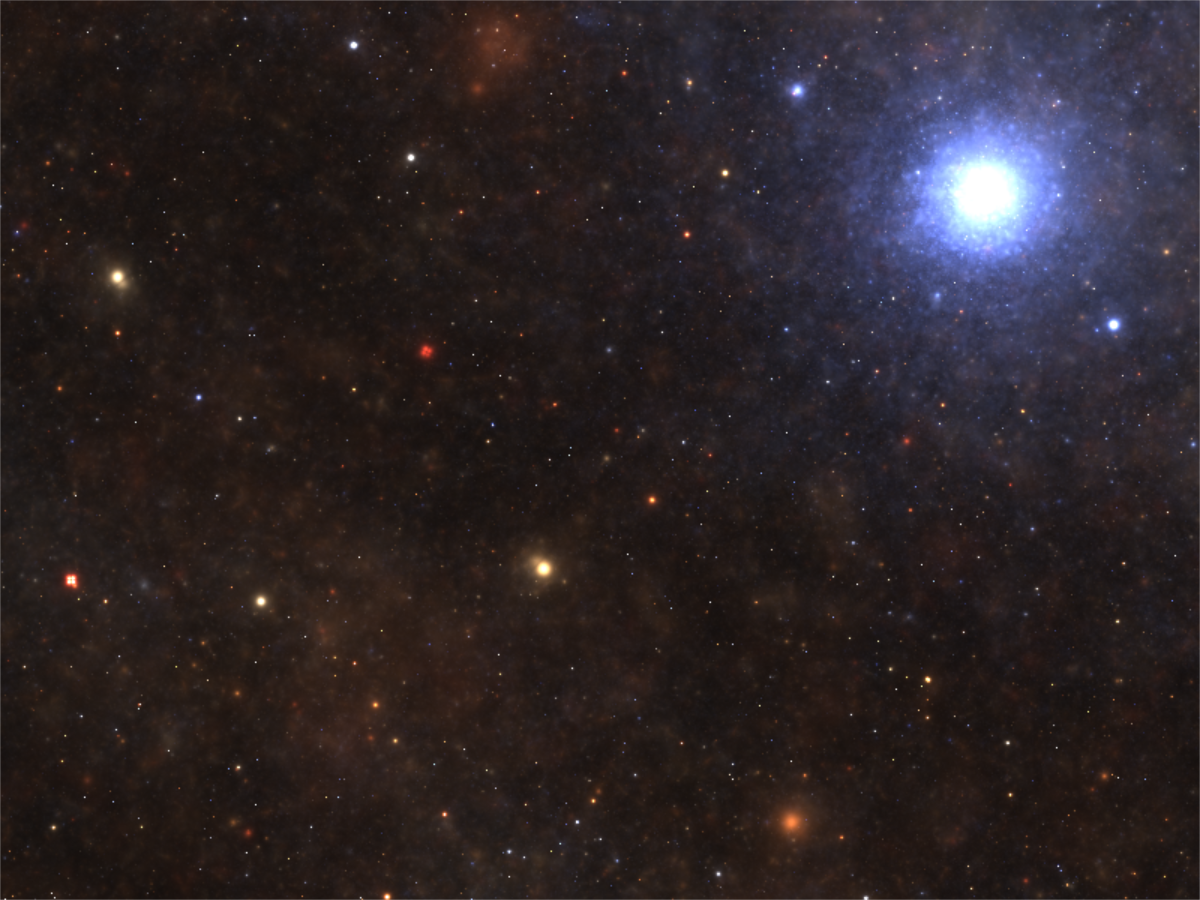
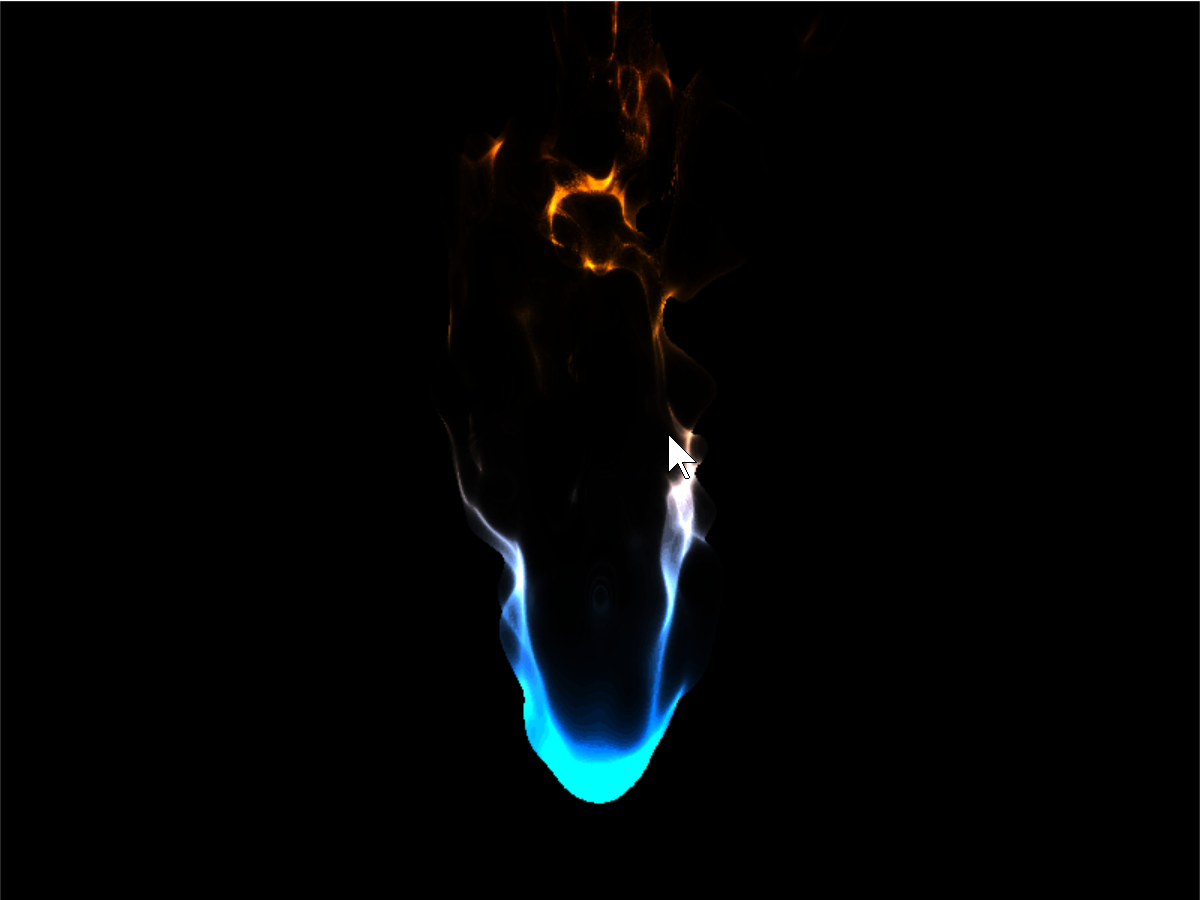
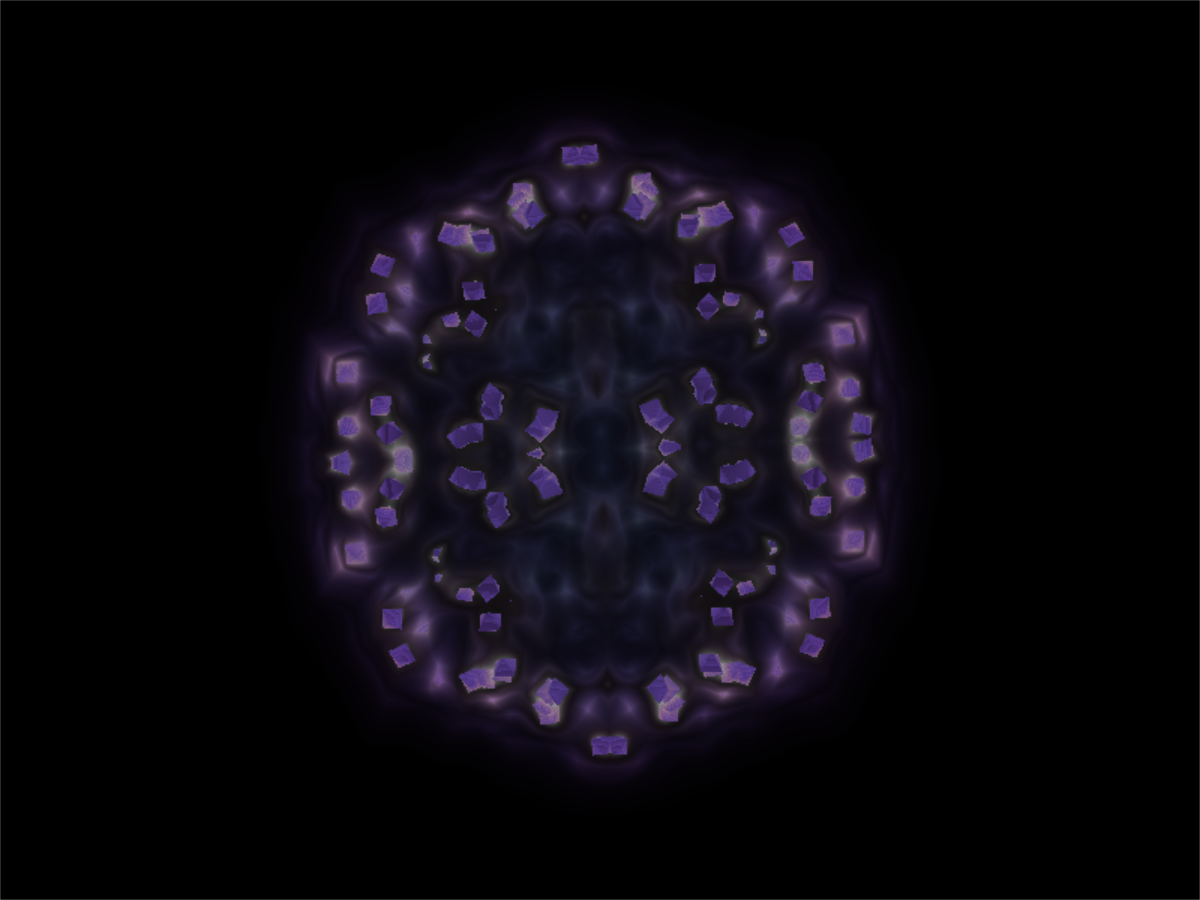
Writing shaders is beyond the scope of this tutorial. Unfortunately, I haven’t found one comprehensive tutorial on how to write a shader. There are several smaller tutorials out there that are good.
Here is one learn-by-example tutorial:
https://www.shadertoy.com/view/Md23DV
Here’s a video tutorial that steps through how to do an explosion: