Flat Text Buttons#
For an introduction the GUI system, see GUI Concepts.
The arcade.gui.UIFlatButton
is a simple button with a text label.
It doesn’t have any three-dimensional look to it.
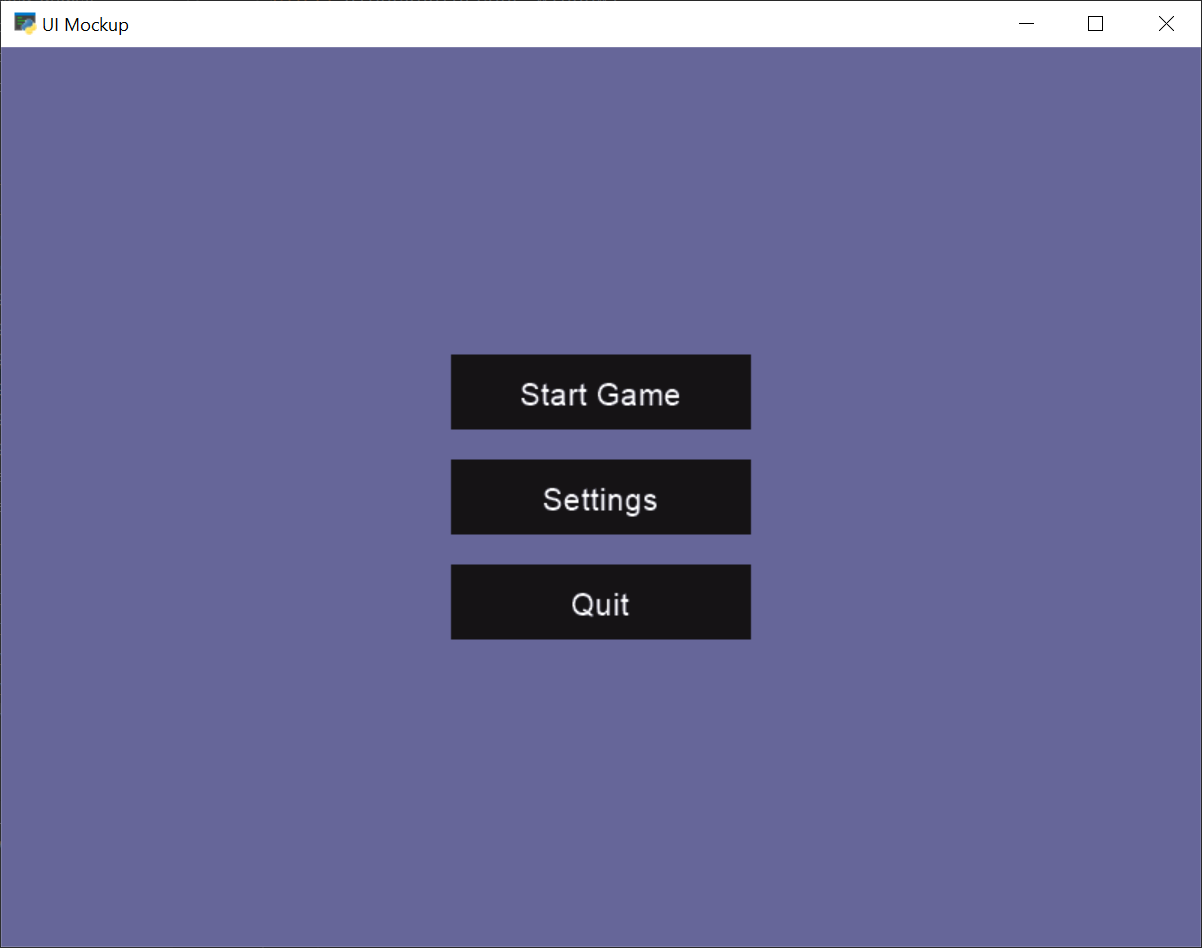
There are three ways to process button click events:
Create a class with a parent class of arcade.UIFlatButton and implement a method called on_click.
Create a button, then set the on_click attribute of that button to equal the function you want to be called.
Create a button. Then use a decorator to specify a method to call when an on_click event occurs for that button.
This code shows each of the three ways above. Code should pick ONE of the three ways and standardize on it though-out the program. Do NOT write code that uses all three ways.
gui_flat_button.py#
1"""
2Example code showing how to create a button,
3and the three ways to process button events.
4
5If Python and Arcade are installed, this example can be run from the command line with:
6python -m arcade.examples.gui_flat_button
7"""
8from __future__ import annotations
9
10import arcade
11import arcade.gui
12
13# --- Method 1 for handling click events,
14# Create a child class.
15import arcade.gui.widgets.buttons
16import arcade.gui.widgets.layout
17
18
19class QuitButton(arcade.gui.widgets.buttons.UIFlatButton):
20 def on_click(self, event: arcade.gui.UIOnClickEvent):
21 arcade.exit()
22
23
24class MyView(arcade.View):
25 def __init__(self):
26 super().__init__()
27
28 # --- Required for all code that uses UI element,
29 # a UIManager to handle the UI.
30 self.ui = arcade.gui.UIManager()
31
32 # Create a vertical BoxGroup to align buttons
33 self.v_box = arcade.gui.widgets.layout.UIBoxLayout(space_between=20)
34
35 # Create the buttons
36 start_button = arcade.gui.widgets.buttons.UIFlatButton(
37 text="Start Game", width=200
38 )
39 self.v_box.add(start_button)
40
41 settings_button = arcade.gui.widgets.buttons.UIFlatButton(
42 text="Settings", width=200
43 )
44 self.v_box.add(settings_button)
45
46 # Again, method 1. Use a child class to handle events.
47 quit_button = QuitButton(text="Quit", width=200)
48 self.v_box.add(quit_button)
49
50 # --- Method 2 for handling click events,
51 # assign self.on_click_start as callback
52 start_button.on_click = self.on_click_start
53
54 # --- Method 3 for handling click events,
55 # use a decorator to handle on_click events
56 @settings_button.event("on_click")
57 def on_click_settings(event):
58 print("Settings:", event)
59
60 # Create a widget to hold the v_box widget, that will center the buttons
61 ui_anchor_layout = arcade.gui.widgets.layout.UIAnchorLayout()
62 ui_anchor_layout.add(child=self.v_box, anchor_x="center_x", anchor_y="center_y")
63
64 self.ui.add(ui_anchor_layout)
65
66 def on_show_view(self):
67 self.window.background_color = arcade.color.DARK_BLUE_GRAY
68 # Enable UIManager when view is shown to catch window events
69 self.ui.enable()
70
71 def on_hide_view(self):
72 # Disable UIManager when view gets inactive
73 self.ui.disable()
74
75 def on_click_start(self, event):
76 print("Start:", event)
77
78 def on_draw(self):
79 self.clear()
80 self.ui.draw()
81
82
83if __name__ == '__main__':
84 window = arcade.Window(800, 600, "UIExample", resizable=True)
85 window.show_view(MyView())
86 window.run()