Step 1 - Install and Open a Window#
Our first step is to make sure everything is installed, and that we can at least get a window open.
Installation#
Make sure Python is installed. Download Python here if you don’t already have it.
Make sure the Arcade library is installed.
You should first setup a virtual environment (venv) and activate it.
Install Arcade with
pip install arcade
.Here are the longer, official Installation Instructions.
I highly recommend using the free community edition of PyCharm as an editor. If you do, see Install Arcade with PyCharm and a Virtual Environment.
Open a Window#
The example below opens up a blank window. Set up a project and get the code
below working. (It is also in the zip file as
01_open_window.py
.)
Note
This is a fixed-size window. It is possible to have a Resizable Window or a Full Screen Example, but there are more interesting things we can do first. Therefore we’ll stick with a fixed-size window for this tutorial.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 | """
Platformer Game
"""
import arcade
# Constants
SCREEN_WIDTH = 1000
SCREEN_HEIGHT = 650
SCREEN_TITLE = "Platformer"
class MyGame(arcade.Window):
"""
Main application class.
"""
def __init__(self):
# Call the parent class and set up the window
super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
arcade.set_background_color(arcade.csscolor.CORNFLOWER_BLUE)
def setup(self):
"""Set up the game here. Call this function to restart the game."""
pass
def on_draw(self):
"""Render the screen."""
self.clear()
# Code to draw the screen goes here
def main():
"""Main function"""
window = MyGame()
window.setup()
arcade.run()
if __name__ == "__main__":
main()
|
You should end up with a window like this:
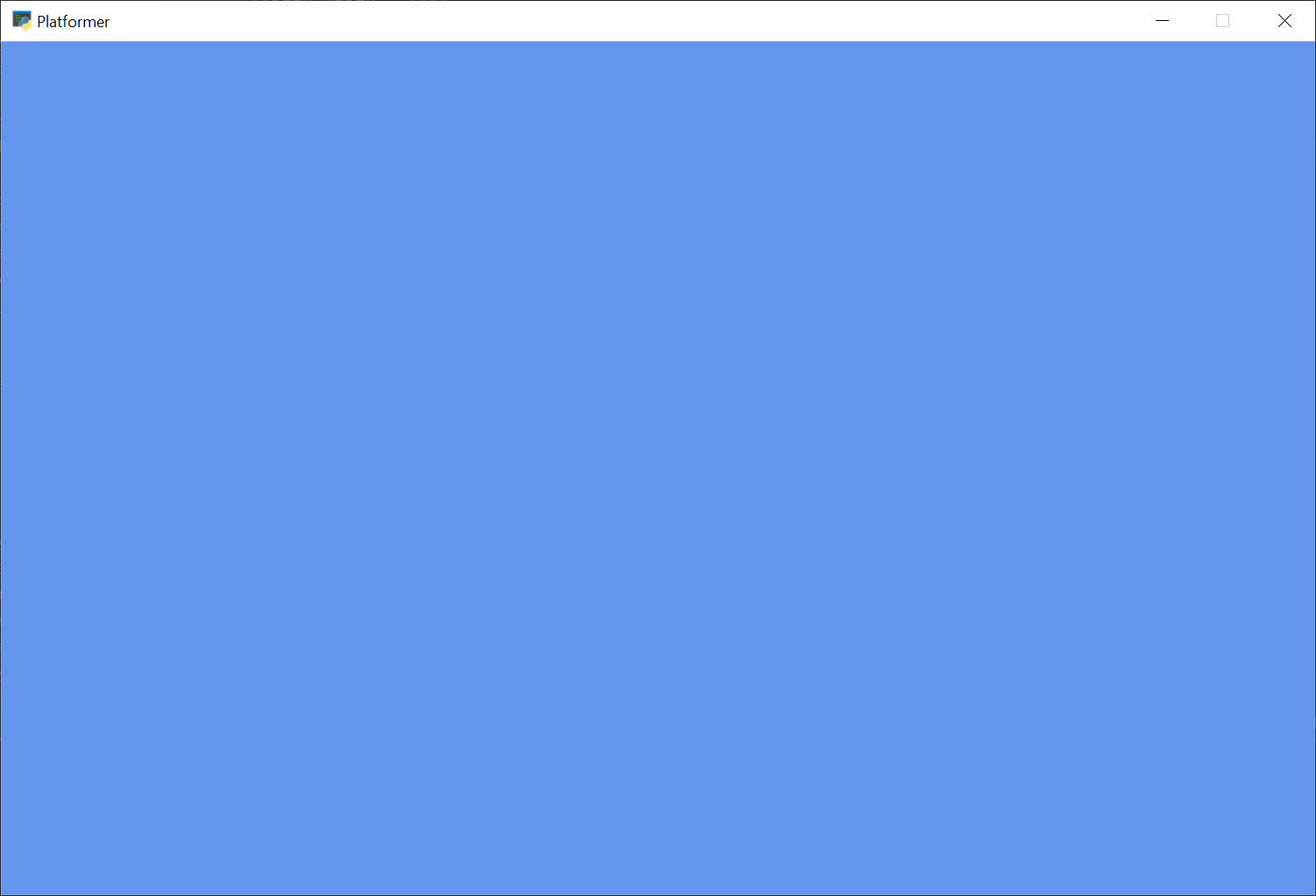
Once you get the code working, figure out how to adjust the code so you can:
Change the screen size
Change the title
Change the background color
See the documentation for arcade.color package
See the documentation for arcade.csscolor package
Look through the documentation for the
arcade.Window
class to get an idea of everything it can do.