Solitaire Tutorial#

This solitaire tutorial takes you though the basics of creating a card game, and doing extensive drag/drop work.
Open a Window#
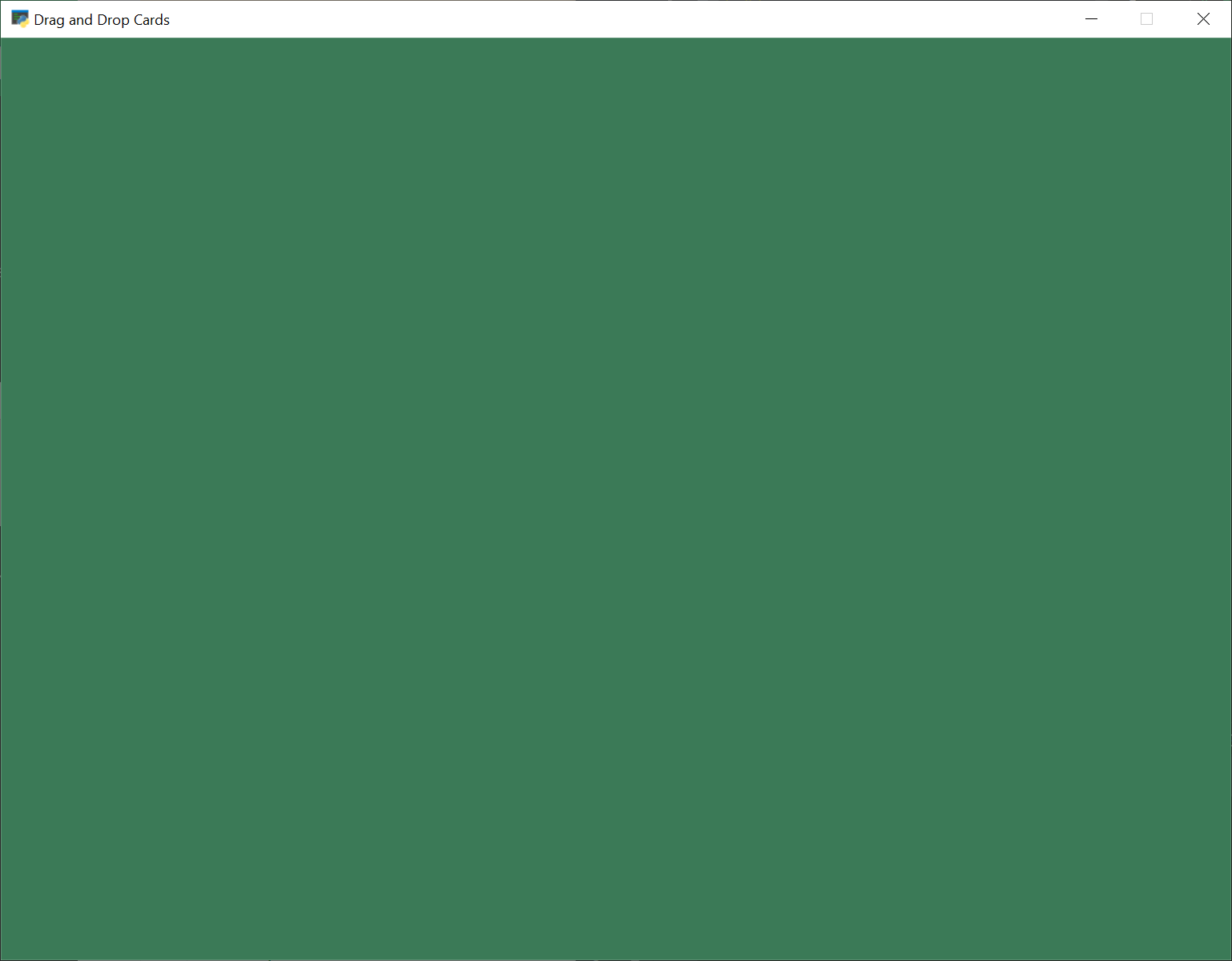
To begin with, let’s start with a program that will use Arcade to open a blank window. The listing below also has stubs for methods we’ll fill in later.
Get started with this code and make sure you can run it. It should pop open a green window.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 | """
Solitaire clone.
"""
import arcade
# Screen title and size
SCREEN_WIDTH = 1024
SCREEN_HEIGHT = 768
SCREEN_TITLE = "Drag and Drop Cards"
class MyGame(arcade.Window):
""" Main application class. """
def __init__(self):
super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
arcade.set_background_color(arcade.color.AMAZON)
def setup(self):
""" Set up the game here. Call this function to restart the game. """
pass
def on_draw(self):
""" Render the screen. """
# Clear the screen
self.clear()
def on_mouse_press(self, x, y, button, key_modifiers):
""" Called when the user presses a mouse button. """
pass
def on_mouse_release(self, x: float, y: float, button: int,
modifiers: int):
""" Called when the user presses a mouse button. """
pass
def on_mouse_motion(self, x: float, y: float, dx: float, dy: float):
""" User moves mouse """
pass
def main():
""" Main function """
window = MyGame()
window.setup()
arcade.run()
if __name__ == "__main__":
main()
|
Create Card Sprites#
Our next step is the create a bunch of sprites, one for each card.
Constants#
First, we’ll create some constants used in positioning the cards, and keeping track of what card is which.
We could just hard-code numbers, but I like to calculate things out. The “mat” will eventually be a square slightly larger than each card that tracks where we can put cards. (A mat where we can put a pile of cards on.)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | # Constants for sizing
CARD_SCALE = 0.6
# How big are the cards?
CARD_WIDTH = 140 * CARD_SCALE
CARD_HEIGHT = 190 * CARD_SCALE
# How big is the mat we'll place the card on?
MAT_PERCENT_OVERSIZE = 1.25
MAT_HEIGHT = int(CARD_HEIGHT * MAT_PERCENT_OVERSIZE)
MAT_WIDTH = int(CARD_WIDTH * MAT_PERCENT_OVERSIZE)
# How much space do we leave as a gap between the mats?
# Done as a percent of the mat size.
VERTICAL_MARGIN_PERCENT = 0.10
HORIZONTAL_MARGIN_PERCENT = 0.10
# The Y of the bottom row (2 piles)
BOTTOM_Y = MAT_HEIGHT / 2 + MAT_HEIGHT * VERTICAL_MARGIN_PERCENT
# The X of where to start putting things on the left side
START_X = MAT_WIDTH / 2 + MAT_WIDTH * HORIZONTAL_MARGIN_PERCENT
# Card constants
CARD_VALUES = ["A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K"]
CARD_SUITS = ["Clubs", "Hearts", "Spades", "Diamonds"]
|
Card Class#
Next up, we’ll create a card class. The card class is a subclass of
arcade.Sprite
. It will have attributes for the suit and value of the
card, and auto-load the image for the card based on that.
We’ll use the entire image as the hit box, so we don’t need to go through the time consuming hit box calculation. Therefore we turn that off. Otherwise loading the sprites would take a long time.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | class Card(arcade.Sprite):
""" Card sprite """
def __init__(self, suit, value, scale=1):
""" Card constructor """
# Attributes for suit and value
self.suit = suit
self.value = value
# Image to use for the sprite when face up
self.image_file_name = f":resources:images/cards/card{self.suit}{self.value}.png"
# Call the parent
super().__init__(self.image_file_name, scale, hit_box_algorithm="None")
|
Creating Cards#
We’ll start by creating an attribute for the SpriteList
that will hold all
the cards in the game.
1 2 3 4 5 6 7 | def __init__(self):
super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
# Sprite list with all the cards, no matter what pile they are in.
self.card_list = None
arcade.set_background_color(arcade.color.AMAZON)
|
In setup
we’ll create the list and the cards. We don’t do this in __init__
because by separating the creation into its own method, we can easily restart the
game by calling setup
.
1 2 3 4 5 6 7 8 9 10 11 12 | def setup(self):
""" Set up the game here. Call this function to restart the game. """
# Sprite list with all the cards, no matter what pile they are in.
self.card_list = arcade.SpriteList()
# Create every card
for card_suit in CARD_SUITS:
for card_value in CARD_VALUES:
card = Card(card_suit, card_value, CARD_SCALE)
card.position = START_X, BOTTOM_Y
self.card_list.append(card)
|
Drawing Cards#
Finally, draw the cards:
1 2 3 4 5 6 7 | def on_draw(self):
""" Render the screen. """
# Clear the screen
self.clear()
# Draw the cards
self.card_list.draw()
|
You should end up with all the cards stacked in the lower-left corner:
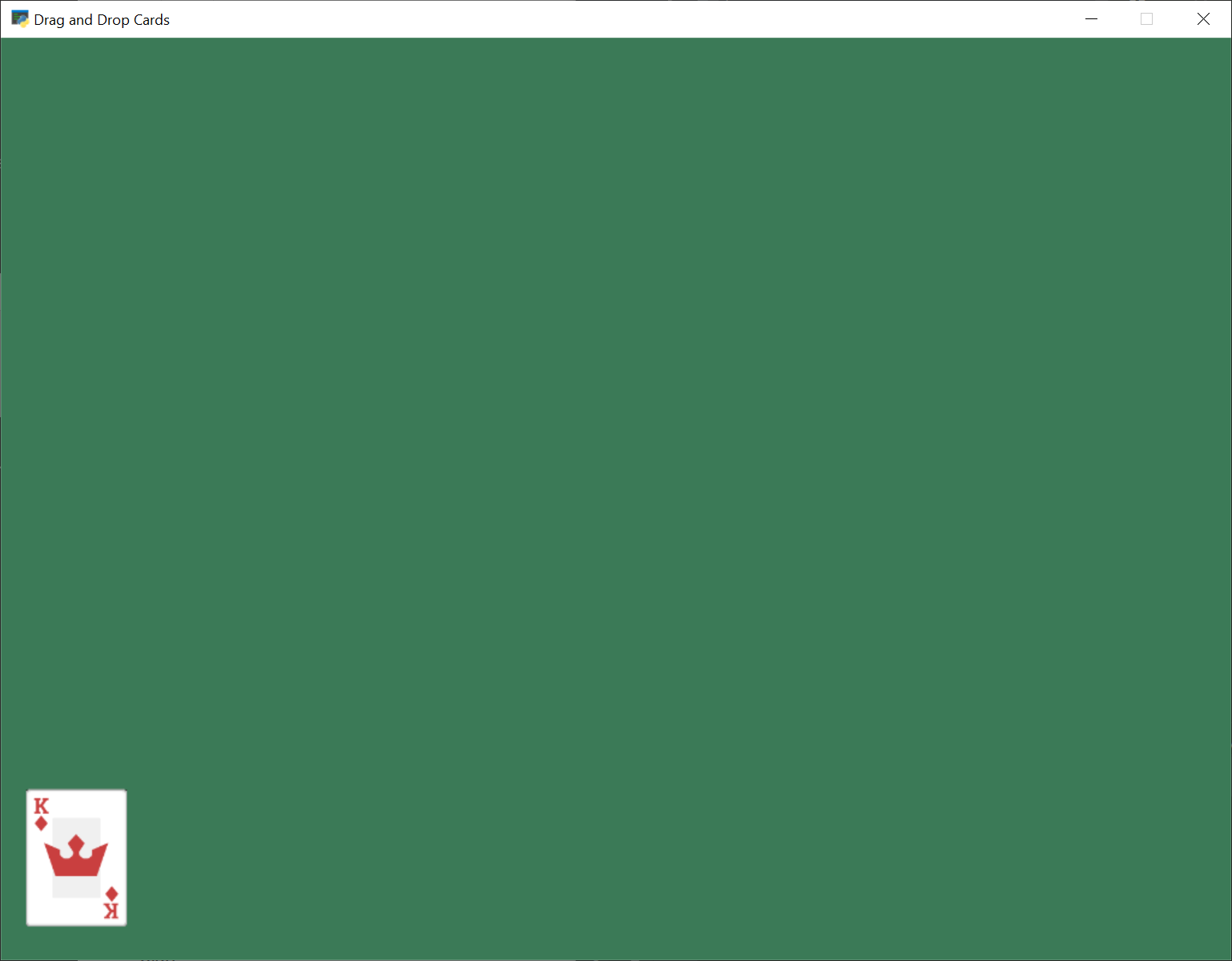
solitaire_02.py Full Listing ← Full listing of where we are right now
solitaire_02.py Diff ← What we changed to get here
Implement Drag and Drop#
Next up, let’s add the ability to pick up, drag, and drop the cards.
Track the Cards#
First, let’s add attributes to track what cards we are moving. Because we can move multiple cards, we’ll keep this as a list. If the user drops the card in an illegal spot, we’ll need to reset the card to its original position. So we’ll also track that.
Create the attributes:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | def __init__(self):
super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
# Sprite list with all the cards, no matter what pile they are in.
self.card_list = None
arcade.set_background_color(arcade.color.AMAZON)
# List of cards we are dragging with the mouse
self.held_cards = None
# Original location of cards we are dragging with the mouse in case
# they have to go back.
self.held_cards_original_position = None
|
Set the initial values (an empty list):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | def setup(self):
""" Set up the game here. Call this function to restart the game. """
# List of cards we are dragging with the mouse
self.held_cards = []
# Original location of cards we are dragging with the mouse in case
# they have to go back.
self.held_cards_original_position = []
# Sprite list with all the cards, no matter what pile they are in.
self.card_list = arcade.SpriteList()
# Create every card
for card_suit in CARD_SUITS:
for card_value in CARD_VALUES:
card = Card(card_suit, card_value, CARD_SCALE)
card.position = START_X, BOTTOM_Y
self.card_list.append(card)
|
Pull Card to Top of Draw Order#
When we click on the card, we’ll want it to be the last card drawn, so it appears on top of all the other cards. Otherwise we might drag a card underneath another card, which would look odd.
1 2 3 4 5 6 | def pull_to_top(self, card: arcade.Sprite):
""" Pull card to top of rendering order (last to render, looks on-top) """
# Remove, and append to the end
self.card_list.remove(card)
self.card_list.append(card)
|
Mouse Moved#
If the user moves the mouse, we’ll move any held cards with it.
1 2 3 4 5 6 7 | def on_mouse_motion(self, x: float, y: float, dx: float, dy: float):
""" User moves mouse """
# If we are holding cards, move them with the mouse
for card in self.held_cards:
card.center_x += dx
card.center_y += dy
|
Mouse Released#
When the user releases the mouse button, we’ll clear the held card list.
1 2 3 4 5 6 7 8 9 10 | def on_mouse_release(self, x: float, y: float, button: int,
modifiers: int):
""" Called when the user presses a mouse button. """
# If we don't have any cards, who cares
if len(self.held_cards) == 0:
return
# We are no longer holding cards
self.held_cards = []
|
Test the Program#
You should now be able to pick up and move cards around the screen. Try it out!
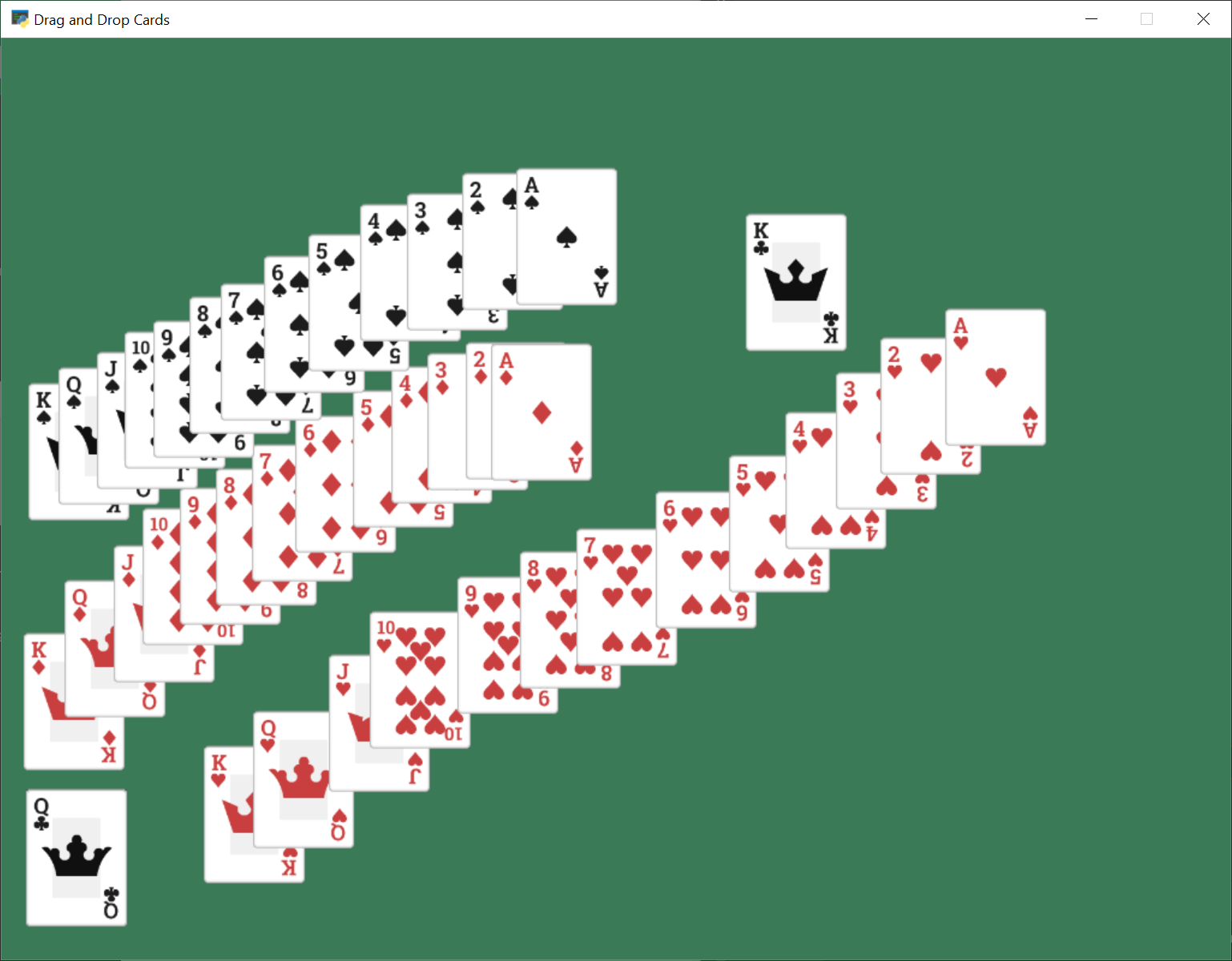
solitaire_03.py Full Listing ← Full listing of where we are right now
solitaire_03.py Diff ← What we changed to get here
Draw Pile Mats#
Next, we’ll create sprites that will act as guides to where the piles of cards go in our game. We’ll create these as sprites, so we can use collision detection to figure out of we are dropping a card on them or not.
Create Constants#
First, we’ll create constants for the middle row of seven piles, and for the top row of four piles. We’ll also create a constant for how far apart each pile should be.
Again, we could hard-code numbers, but I like calculating them so I can change the scale easily.
1 2 3 4 5 6 7 8 | # The Y of the top row (4 piles)
TOP_Y = SCREEN_HEIGHT - MAT_HEIGHT / 2 - MAT_HEIGHT * VERTICAL_MARGIN_PERCENT
# The Y of the middle row (7 piles)
MIDDLE_Y = TOP_Y - MAT_HEIGHT - MAT_HEIGHT * VERTICAL_MARGIN_PERCENT
# How far apart each pile goes
X_SPACING = MAT_WIDTH + MAT_WIDTH * HORIZONTAL_MARGIN_PERCENT
|
Create Mat Sprites#
Create an attribute for the mat sprite list:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | def __init__(self):
super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
# Sprite list with all the cards, no matter what pile they are in.
self.card_list = None
arcade.set_background_color(arcade.color.AMAZON)
# List of cards we are dragging with the mouse
self.held_cards = None
# Original location of cards we are dragging with the mouse in case
# they have to go back.
self.held_cards_original_position = None
# Sprite list with all the mats tha cards lay on.
self.pile_mat_list = None
|
Then create the mat sprites in the setup
method
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 | def setup(self):
""" Set up the game here. Call this function to restart the game. """
# List of cards we are dragging with the mouse
self.held_cards = []
# Original location of cards we are dragging with the mouse in case
# they have to go back.
self.held_cards_original_position = []
# --- Create the mats the cards go on.
# Sprite list with all the mats tha cards lay on.
self.pile_mat_list: arcade.SpriteList = arcade.SpriteList()
# Create the mats for the bottom face down and face up piles
pile = arcade.SpriteSolidColor(MAT_WIDTH, MAT_HEIGHT, arcade.csscolor.DARK_OLIVE_GREEN)
pile.position = START_X, BOTTOM_Y
self.pile_mat_list.append(pile)
pile = arcade.SpriteSolidColor(MAT_WIDTH, MAT_HEIGHT, arcade.csscolor.DARK_OLIVE_GREEN)
pile.position = START_X + X_SPACING, BOTTOM_Y
self.pile_mat_list.append(pile)
# Create the seven middle piles
for i in range(7):
pile = arcade.SpriteSolidColor(MAT_WIDTH, MAT_HEIGHT, arcade.csscolor.DARK_OLIVE_GREEN)
pile.position = START_X + i * X_SPACING, MIDDLE_Y
self.pile_mat_list.append(pile)
# Create the top "play" piles
for i in range(4):
pile = arcade.SpriteSolidColor(MAT_WIDTH, MAT_HEIGHT, arcade.csscolor.DARK_OLIVE_GREEN)
pile.position = START_X + i * X_SPACING, TOP_Y
self.pile_mat_list.append(pile)
# Sprite list with all the cards, no matter what pile they are in.
self.card_list = arcade.SpriteList()
# Create every card
for card_suit in CARD_SUITS:
for card_value in CARD_VALUES:
card = Card(card_suit, card_value, CARD_SCALE)
card.position = START_X, BOTTOM_Y
self.card_list.append(card)
|
Draw Mat Sprites#
Finally, the mats aren’t going to display if we don’t draw them:
1 2 3 4 5 6 7 8 9 10 | def on_draw(self):
""" Render the screen. """
# Clear the screen
self.clear()
# Draw the mats the cards go on to
self.pile_mat_list.draw()
# Draw the cards
self.card_list.draw()
|
Test the Program#
Run the program, and see if the mats appear:
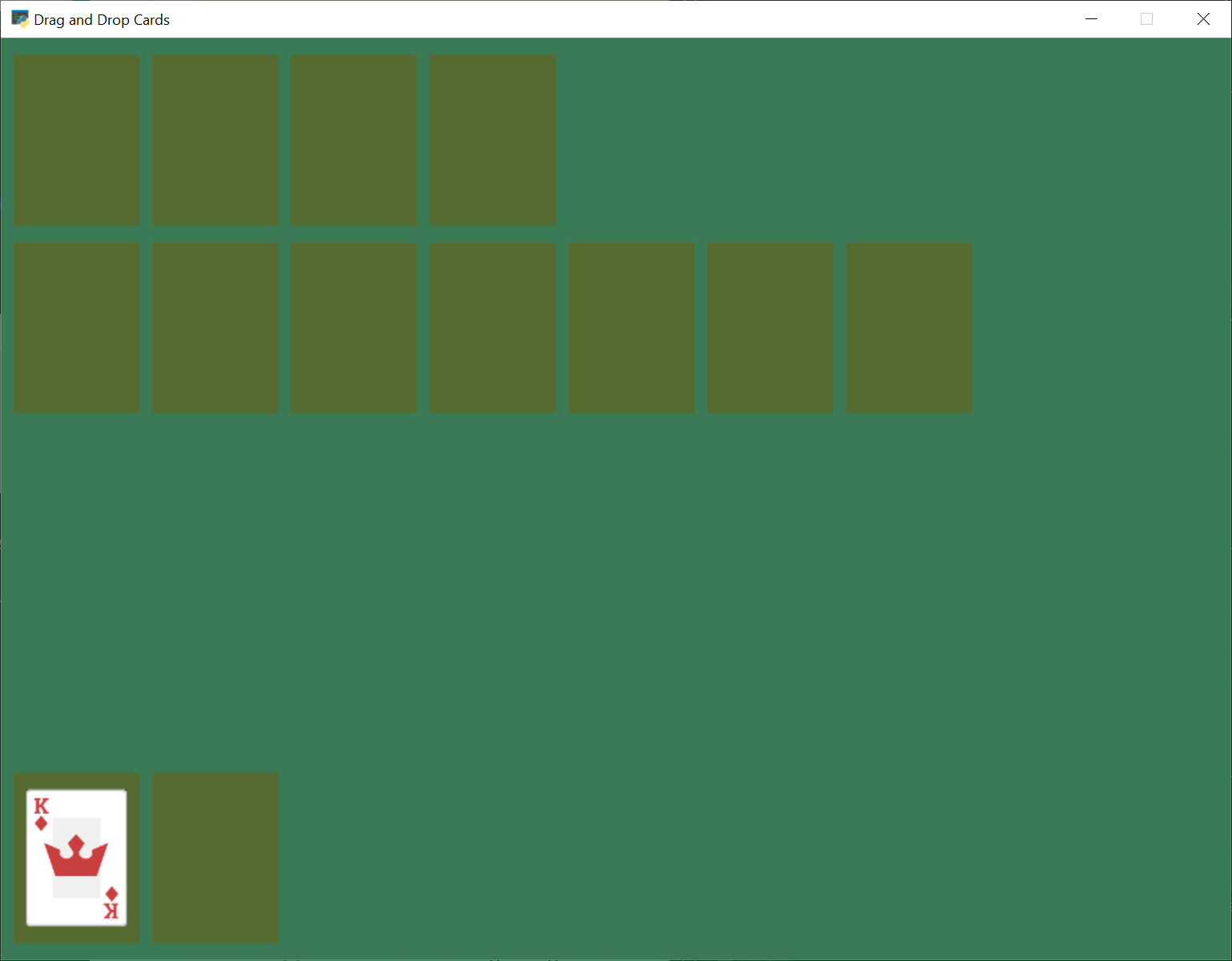
solitaire_04.py Full Listing ← Full listing of where we are right now
solitaire_04.py Diff ← What we changed to get here
Snap Cards to Piles#
Right now, you can drag the cards anywhere. They don’t have to go onto a pile. Let’s add code that “snaps” the card onto a pile. If we don’t drop on a pile, let’s reset back to the original location.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | def on_mouse_release(self, x: float, y: float, button: int,
modifiers: int):
""" Called when the user presses a mouse button. """
# If we don't have any cards, who cares
if len(self.held_cards) == 0:
return
# Find the closest pile, in case we are in contact with more than one
pile, distance = arcade.get_closest_sprite(self.held_cards[0], self.pile_mat_list)
reset_position = True
# See if we are in contact with the closest pile
if arcade.check_for_collision(self.held_cards[0], pile):
# For each held card, move it to the pile we dropped on
for i, dropped_card in enumerate(self.held_cards):
# Move cards to proper position
dropped_card.position = pile.center_x, pile.center_y
# Success, don't reset position of cards
reset_position = False
# Release on top play pile? And only one card held?
if reset_position:
# Where-ever we were dropped, it wasn't valid. Reset the each card's position
# to its original spot.
for pile_index, card in enumerate(self.held_cards):
card.position = self.held_cards_original_position[pile_index]
# We are no longer holding cards
self.held_cards = []
|
solitaire_05.py Full Listing ← Full listing of where we are right now
solitaire_05.py Diff ← What we changed to get here
Shuffle the Cards#
Having all the cards in order is boring. Let’s shuffle them in the setup
method:
1 2 3 4 | # Shuffle the cards
for pos1 in range(len(self.card_list)):
pos2 = random.randrange(len(self.card_list))
self.card_list.swap(pos1, pos2)
|
Don’t forget to import random
at the top.
Run your program and make sure you can move cards around.
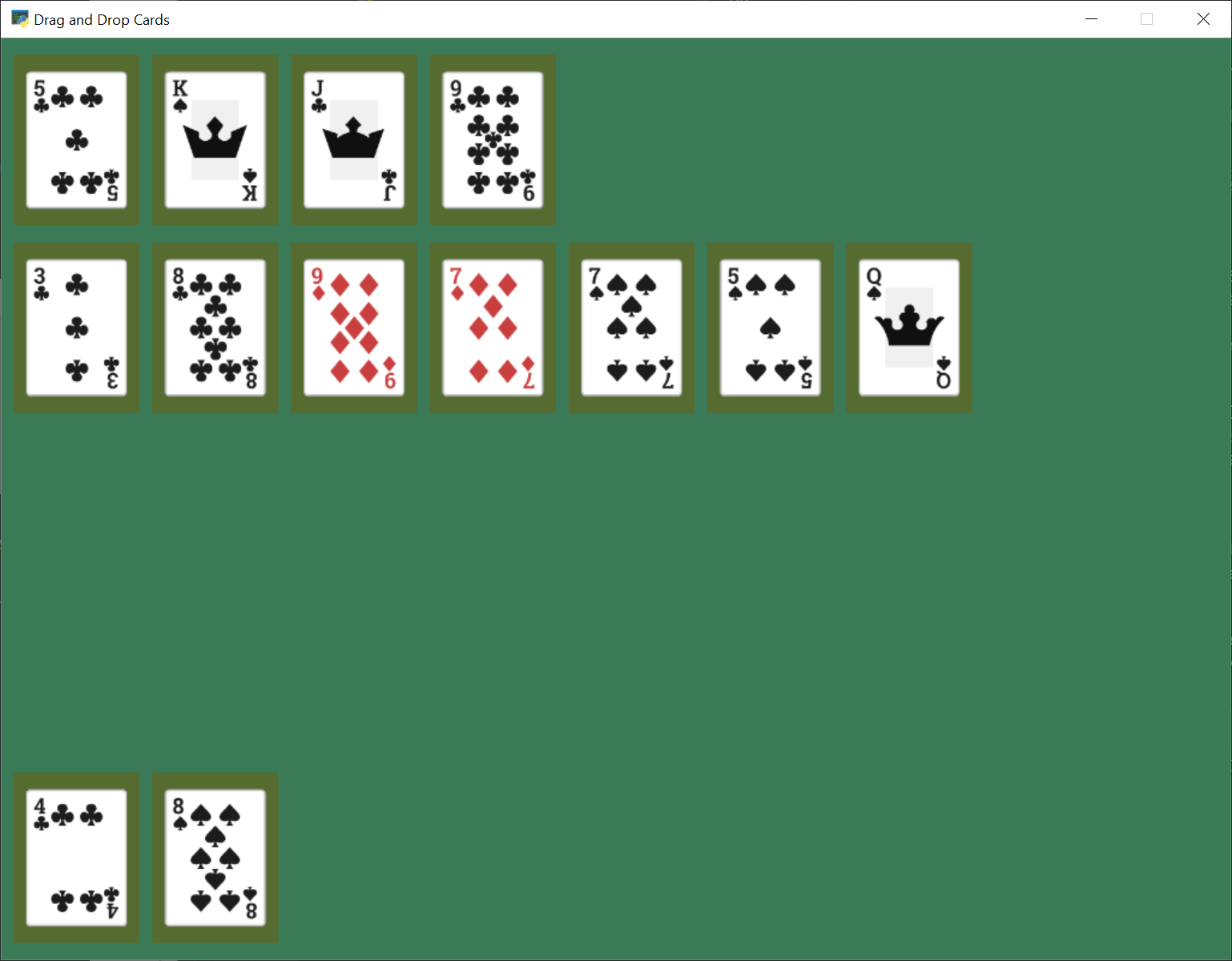
solitaire_06.py Full Listing ← Full listing of where we are right now
solitaire_06.py Diff ← What we changed to get here
Track Card Piles#
Right now we are moving the cards around. But it isn’t easy to figure out what card is in which pile. We could check by position, but then we start fanning the cards out, that will be very difficult.
Therefore we will keep a separate list for each pile of cards. When we move a card we need to move the position, and switch which list it is in.
Add New Constants#
To start with, let’s add some constants for each pile:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | # If we fan out cards stacked on each other, how far apart to fan them?
CARD_VERTICAL_OFFSET = CARD_HEIGHT * CARD_SCALE * 0.3
# Constants that represent "what pile is what" for the game
PILE_COUNT = 13
BOTTOM_FACE_DOWN_PILE = 0
BOTTOM_FACE_UP_PILE = 1
PLAY_PILE_1 = 2
PLAY_PILE_2 = 3
PLAY_PILE_3 = 4
PLAY_PILE_4 = 5
PLAY_PILE_5 = 6
PLAY_PILE_6 = 7
PLAY_PILE_7 = 8
TOP_PILE_1 = 9
TOP_PILE_2 = 10
TOP_PILE_3 = 11
TOP_PILE_4 = 12
|
Create the Pile Lists#
Then in our __init__
add a variable to track the piles:
1 2 | # Create a list of lists, each holds a pile of cards.
self.piles = None
|
In the setup
method, create a list for each pile. Then, add all the cards
to the face-down deal pile. (Later, we’ll add support for face-down cards.
Yes, right now all the cards in the face down pile are up.)
1 2 3 4 5 6 | # Create a list of lists, each holds a pile of cards.
self.piles = [[] for _ in range(PILE_COUNT)]
# Put all the cards in the bottom face-down pile
for card in self.card_list:
self.piles[BOTTOM_FACE_DOWN_PILE].append(card)
|
Card Pile Management Methods#
Next, we need some convenience methods we’ll use elsewhere.
First, given a card, return the index of which pile that card belongs to:
1 2 3 4 5 | def get_pile_for_card(self, card):
""" What pile is this card in? """
for index, pile in enumerate(self.piles):
if card in pile:
return index
|
Next, remove a card from whatever pile it happens to be in.
1 2 3 4 5 6 | def remove_card_from_pile(self, card):
""" Remove card from whatever pile it was in. """
for pile in self.piles:
if card in pile:
pile.remove(card)
break
|
Finally, move a card from one pile to another.
1 2 3 4 | def move_card_to_new_pile(self, card, pile_index):
""" Move the card to a new pile """
self.remove_card_from_pile(card)
self.piles[pile_index].append(card)
|
Dropping the Card#
Next, we need to modify what happens when we release the mouse.
First, see if we release it onto the same pile it came from. If so, just reset the card back to its original location.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | def on_mouse_release(self, x: float, y: float, button: int,
modifiers: int):
""" Called when the user presses a mouse button. """
# If we don't have any cards, who cares
if len(self.held_cards) == 0:
return
# Find the closest pile, in case we are in contact with more than one
pile, distance = arcade.get_closest_sprite(self.held_cards[0], self.pile_mat_list)
reset_position = True
# See if we are in contact with the closest pile
if arcade.check_for_collision(self.held_cards[0], pile):
# What pile is it?
pile_index = self.pile_mat_list.index(pile)
# Is it the same pile we came from?
if pile_index == self.get_pile_for_card(self.held_cards[0]):
# If so, who cares. We'll just reset our position.
pass
|
What if it is on a middle play pile? Ugh, that’s a bit complicated. If the mat is empty, we need to place it in the middle of the mat. If there are cards on the mat, we need to offset the card so we can see a spread of cards.
While we can only pick up one card at a time right now, we need to support dropping multiple cards for once we support multiple card carries.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | # Is it on a middle play pile?
elif PLAY_PILE_1 <= pile_index <= PLAY_PILE_7:
# Are there already cards there?
if len(self.piles[pile_index]) > 0:
# Move cards to proper position
top_card = self.piles[pile_index][-1]
for i, dropped_card in enumerate(self.held_cards):
dropped_card.position = top_card.center_x, \
top_card.center_y - CARD_VERTICAL_OFFSET * (i + 1)
else:
# Are there no cards in the middle play pile?
for i, dropped_card in enumerate(self.held_cards):
# Move cards to proper position
dropped_card.position = pile.center_x, \
pile.center_y - CARD_VERTICAL_OFFSET * i
for card in self.held_cards:
# Cards are in the right position, but we need to move them to the right list
self.move_card_to_new_pile(card, pile_index)
# Success, don't reset position of cards
reset_position = False
|
What if it is released on a top play pile? Make sure that we only have one card we are holding. We don’t want to drop a stack up top. Then move the card to that pile.
1 2 3 4 5 6 7 8 9 | # Release on top play pile? And only one card held?
elif TOP_PILE_1 <= pile_index <= TOP_PILE_4 and len(self.held_cards) == 1:
# Move position of card to pile
self.held_cards[0].position = pile.position
# Move card to card list
for card in self.held_cards:
self.move_card_to_new_pile(card, pile_index)
reset_position = False
|
If the move is invalid, we need to reset all held cards to their initial location.
1 2 3 4 5 6 7 8 | if reset_position:
# Where-ever we were dropped, it wasn't valid. Reset the each card's position
# to its original spot.
for pile_index, card in enumerate(self.held_cards):
card.position = self.held_cards_original_position[pile_index]
# We are no longer holding cards
self.held_cards = []
|
Test#
Test out your program, and see if the cards are being fanned out properly.
Note
The code isn’t enforcing any game rules. You can stack cards in any order. Also, with long stacks of cards, you still have to drop the card on the mat. This is counter-intuitive when the stack of cards extends downwards past the mat.
We leave the solutions to these issues as an exercise for the reader.
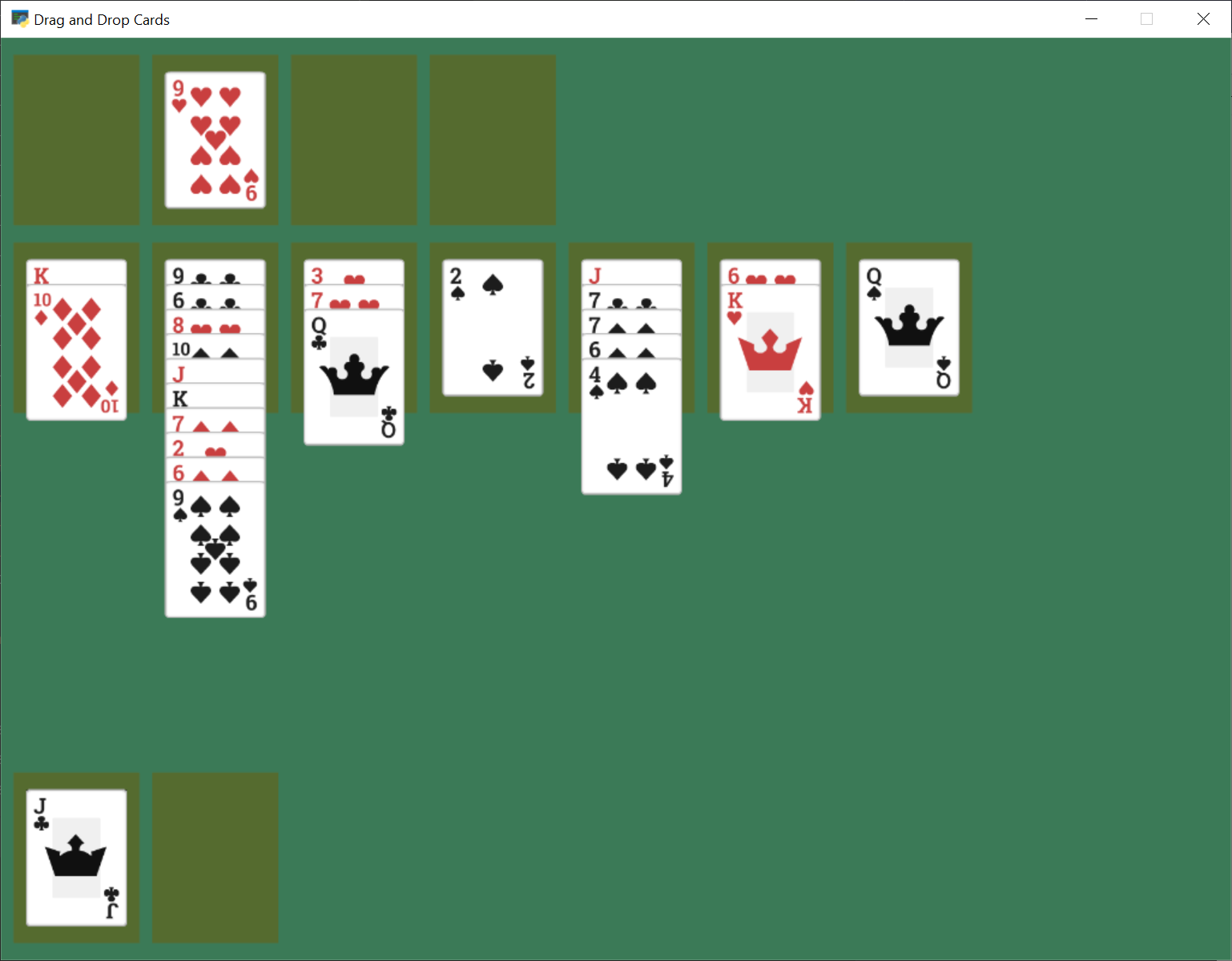
solitaire_07.py Full Listing ← Full listing of where we are right now
solitaire_07.py Diff ← What we changed to get here
Pick Up Card Stacks#
How do we pick up a whole stack of cards? When the mouse is pressed, we need to figure out what pile the card is in.
Next, look at where in the pile the card is that we clicked on. If there are any cards later on on the pile, we want to pick up those cards too. Add them to the list.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | def on_mouse_press(self, x, y, button, key_modifiers):
""" Called when the user presses a mouse button. """
# Get list of cards we've clicked on
cards = arcade.get_sprites_at_point((x, y), self.card_list)
# Have we clicked on a card?
if len(cards) > 0:
# Might be a stack of cards, get the top one
primary_card = cards[-1]
# Figure out what pile the card is in
pile_index = self.get_pile_for_card(primary_card)
# All other cases, grab the face-up card we are clicking on
self.held_cards = [primary_card]
# Save the position
self.held_cards_original_position = [self.held_cards[0].position]
# Put on top in drawing order
self.pull_to_top(self.held_cards[0])
# Is this a stack of cards? If so, grab the other cards too
card_index = self.piles[pile_index].index(primary_card)
for i in range(card_index + 1, len(self.piles[pile_index])):
card = self.piles[pile_index][i]
self.held_cards.append(card)
self.held_cards_original_position.append(card.position)
self.pull_to_top(card)
|
After this, you should be able to pick up a stack of cards from the middle piles with the mouse and move them around.
solitaire_08.py Full Listing ← Full listing of where we are right now
solitaire_08.py Diff ← What we changed to get here
Deal Out Cards#
We can deal the cards into the seven middle piles by adding some code to the
setup
method. We need to change the list each card is part of, along with
its position.
1 2 3 4 5 6 7 8 9 10 11 12 13 | # - Pull from that pile into the middle piles, all face-down
# Loop for each pile
for pile_no in range(PLAY_PILE_1, PLAY_PILE_7 + 1):
# Deal proper number of cards for that pile
for j in range(pile_no - PLAY_PILE_1 + 1):
# Pop the card off the deck we are dealing from
card = self.piles[BOTTOM_FACE_DOWN_PILE].pop()
# Put in the proper pile
self.piles[pile_no].append(card)
# Move card to same position as pile we just put it in
card.position = self.pile_mat_list[pile_no].position
# Put on top in draw order
self.pull_to_top(card)
|
solitaire_09.py Full Listing ← Full listing of where we are right now
solitaire_09.py Diff ← What we changed to get here
Face Down Cards#
We don’t play solitaire with all the cards facing up, so let’s add face-down support to our game.
New Constants#
First define a constant for what image to use when face-down.
1 2 | # Face down image
FACE_DOWN_IMAGE = ":resources:images/cards/cardBack_red2.png"
|
Updates to Card Class#
Next, default each card in the Card
class to be face up. Also, let’s add
methods to flip the card up or down.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | class Card(arcade.Sprite):
""" Card sprite """
def __init__(self, suit, value, scale=1):
""" Card constructor """
# Attributes for suit and value
self.suit = suit
self.value = value
# Image to use for the sprite when face up
self.image_file_name = f":resources:images/cards/card{self.suit}{self.value}.png"
self.is_face_up = False
super().__init__(FACE_DOWN_IMAGE, scale, hit_box_algorithm="None")
def face_down(self):
""" Turn card face-down """
self.texture = arcade.load_texture(FACE_DOWN_IMAGE)
self.is_face_up = False
def face_up(self):
""" Turn card face-up """
self.texture = arcade.load_texture(self.image_file_name)
self.is_face_up = True
@property
def is_face_down(self):
""" Is this card face down? """
return not self.is_face_up
|
Flip Up Cards On Middle Seven Piles#
Right now every card is face down. Let’s update the setup
method so the
top cards in the middle seven piles are face up.
1 2 3 | # Flip up the top cards
for i in range(PLAY_PILE_1, PLAY_PILE_7 + 1):
self.piles[i][-1].face_up()
|
Flip Up Cards When Clicked#
When we click on a card that is face down, instead of picking it up, let’s flip it over:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | def on_mouse_press(self, x, y, button, key_modifiers):
""" Called when the user presses a mouse button. """
# Get list of cards we've clicked on
cards = arcade.get_sprites_at_point((x, y), self.card_list)
# Have we clicked on a card?
if len(cards) > 0:
# Might be a stack of cards, get the top one
primary_card = cards[-1]
assert isinstance(primary_card, Card)
# Figure out what pile the card is in
pile_index = self.get_pile_for_card(primary_card)
if primary_card.is_face_down:
# Is the card face down? In one of those middle 7 piles? Then flip up
primary_card.face_up()
else:
# All other cases, grab the face-up card we are clicking on
self.held_cards = [primary_card]
# Save the position
self.held_cards_original_position = [self.held_cards[0].position]
# Put on top in drawing order
self.pull_to_top(self.held_cards[0])
# Is this a stack of cards? If so, grab the other cards too
card_index = self.piles[pile_index].index(primary_card)
for i in range(card_index + 1, len(self.piles[pile_index])):
card = self.piles[pile_index][i]
self.held_cards.append(card)
self.held_cards_original_position.append(card.position)
self.pull_to_top(card)
|
Test#
Try out your program. As you move cards around, you should see face down cards as well, and be able to flip them over.
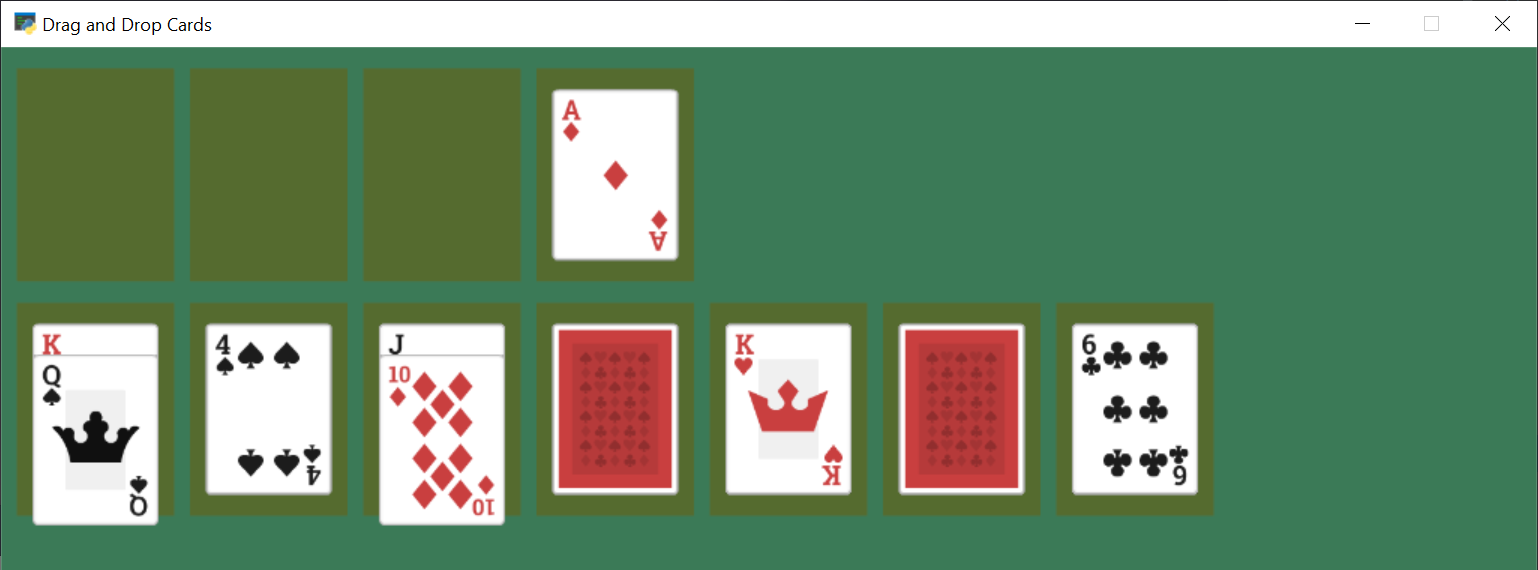
solitaire_10.py Full Listing ← Full listing of where we are right now
solitaire_10.py Diff ← What we changed to get here
Restart Game#
We can add the ability to restart are game any type we press the ‘R’ key:
1 2 3 4 5 | def on_key_press(self, symbol: int, modifiers: int):
""" User presses key """
if symbol == arcade.key.R:
# Restart
self.setup()
|
Flip Three From Draw Pile#
The draw pile at the bottom of our screen doesn’t work right yet. When we click on it, we need it to flip three cards to the bottom-right pile. Also, if the have gone through all the cards in the pile, we need to reset the pile so we can go through it again.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 | def on_mouse_press(self, x, y, button, key_modifiers):
""" Called when the user presses a mouse button. """
# Get list of cards we've clicked on
cards = arcade.get_sprites_at_point((x, y), self.card_list)
# Have we clicked on a card?
if len(cards) > 0:
# Might be a stack of cards, get the top one
primary_card = cards[-1]
assert isinstance(primary_card, Card)
# Figure out what pile the card is in
pile_index = self.get_pile_for_card(primary_card)
# Are we clicking on the bottom deck, to flip three cards?
if pile_index == BOTTOM_FACE_DOWN_PILE:
# Flip three cards
for i in range(3):
# If we ran out of cards, stop
if len(self.piles[BOTTOM_FACE_DOWN_PILE]) == 0:
break
# Get top card
card = self.piles[BOTTOM_FACE_DOWN_PILE][-1]
# Flip face up
card.face_up()
# Move card position to bottom-right face up pile
card.position = self.pile_mat_list[BOTTOM_FACE_UP_PILE].position
# Remove card from face down pile
self.piles[BOTTOM_FACE_DOWN_PILE].remove(card)
# Move card to face up list
self.piles[BOTTOM_FACE_UP_PILE].append(card)
# Put on top draw-order wise
self.pull_to_top(card)
elif primary_card.is_face_down:
# Is the card face down? In one of those middle 7 piles? Then flip up
primary_card.face_up()
else:
# All other cases, grab the face-up card we are clicking on
self.held_cards = [primary_card]
# Save the position
self.held_cards_original_position = [self.held_cards[0].position]
# Put on top in drawing order
self.pull_to_top(self.held_cards[0])
# Is this a stack of cards? If so, grab the other cards too
card_index = self.piles[pile_index].index(primary_card)
for i in range(card_index + 1, len(self.piles[pile_index])):
card = self.piles[pile_index][i]
self.held_cards.append(card)
self.held_cards_original_position.append(card.position)
self.pull_to_top(card)
else:
# Click on a mat instead of a card?
mats = arcade.get_sprites_at_point((x, y), self.pile_mat_list)
if len(mats) > 0:
mat = mats[0]
mat_index = self.pile_mat_list.index(mat)
# Is it our turned over flip mat? and no cards on it?
if mat_index == BOTTOM_FACE_DOWN_PILE and len(self.piles[BOTTOM_FACE_DOWN_PILE]) == 0:
# Flip the deck back over so we can restart
temp_list = self.piles[BOTTOM_FACE_UP_PILE].copy()
for card in reversed(temp_list):
card.face_down()
self.piles[BOTTOM_FACE_UP_PILE].remove(card)
self.piles[BOTTOM_FACE_DOWN_PILE].append(card)
card.position = self.pile_mat_list[BOTTOM_FACE_DOWN_PILE].position
|
Test#
Now we’ve got a basic working solitaire game! Try it out!

solitaire_11.py Full Listing ← Full listing of where we are right now
solitaire_11.py Diff ← What we changed to get here
Conclusion#
There’s a lot more that could be added to this game, such as enforcing rules, adding animation to ‘slide’ a dropped card to its position, sound, better graphics, and more. Or this could be adapted to a different card game.
Hopefully this is enough to get you started on your own game.