Different Levels of Clearing Coins#
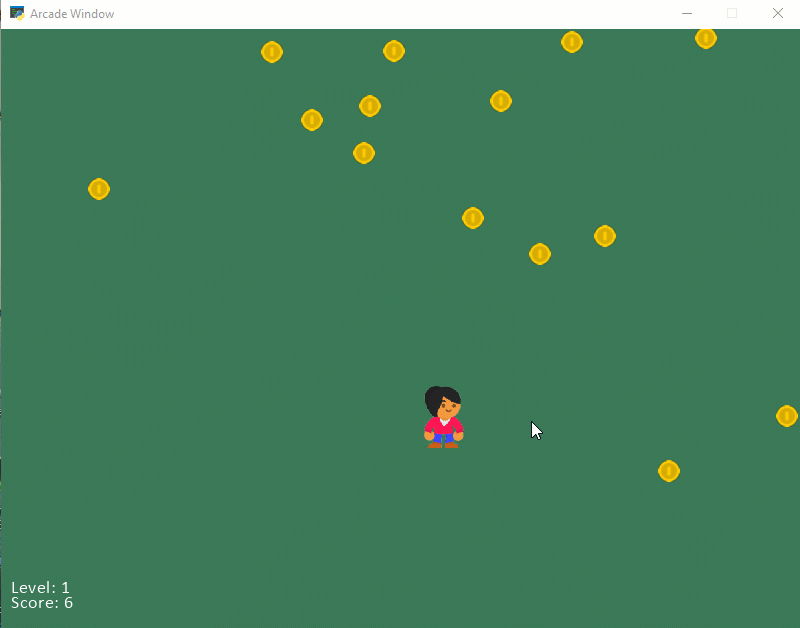
sprite_collect_coins_diff_levels.py#
1"""
2Sprite Collect Coins with Different Levels
3
4Simple program to show basic sprite usage.
5
6Artwork from https://kenney.nl
7
8If Python and Arcade are installed, this example can be run from the command line with:
9python -m arcade.examples.sprite_collect_coins_diff_levels
10"""
11
12import random
13import arcade
14
15SPRITE_SCALING = 0.5
16
17SCREEN_WIDTH = 800
18SCREEN_HEIGHT = 600
19SCREEN_TITLE = "Sprite Collect Coins with Different Levels Example"
20
21
22class FallingCoin(arcade.Sprite):
23 """ Simple sprite that falls down """
24
25 def update(self):
26 """ Move the coin """
27
28 # Fall down
29 self.center_y -= 2
30
31 # Did we go off the screen? If so, pop back to the top.
32 if self.top < 0:
33 self.bottom = SCREEN_HEIGHT
34
35
36class RisingCoin(arcade.Sprite):
37 """ Simple sprite that falls up """
38
39 def update(self):
40 """ Move the coin """
41
42 # Move up
43 self.center_y += 2
44
45 # Did we go off the screen? If so, pop back to the bottom.
46 if self.bottom > SCREEN_HEIGHT:
47 self.top = 0
48
49
50class MyGame(arcade.Window):
51 """
52 Main application class.
53 """
54
55 def __init__(self, width, height, title):
56 """ Initialize """
57
58 # Call the parent class initializer
59 super().__init__(width, height, title)
60
61 # Variables that will hold sprite lists
62 self.player_list = None
63 self.coin_list = None
64
65 # Set up the player info
66 self.player_sprite = None
67 self.score = 0
68
69 self.level = 1
70
71 # Don't show the mouse cursor
72 self.set_mouse_visible(False)
73
74 # Set the background color
75 self.background_color = arcade.color.AMAZON
76
77 def level_1(self):
78 for i in range(20):
79
80 # Create the coin instance
81 coin = arcade.Sprite(":resources:images/items/coinGold.png", scale=SPRITE_SCALING / 3)
82
83 # Position the coin
84 coin.center_x = random.randrange(SCREEN_WIDTH)
85 coin.center_y = random.randrange(SCREEN_HEIGHT)
86
87 # Add the coin to the lists
88 self.coin_list.append(coin)
89
90 def level_2(self):
91 for i in range(30):
92
93 # Create the coin instance
94 coin = FallingCoin(":resources:images/items/coinBronze.png", scale=SPRITE_SCALING / 2)
95
96 # Position the coin
97 coin.center_x = random.randrange(SCREEN_WIDTH)
98 coin.center_y = random.randrange(SCREEN_HEIGHT, SCREEN_HEIGHT * 2)
99
100 # Add the coin to the lists
101 self.coin_list.append(coin)
102
103 def level_3(self):
104 for i in range(30):
105
106 # Create the coin instance
107 coin = RisingCoin(":resources:images/items/coinSilver.png", scale=SPRITE_SCALING / 2)
108
109 # Position the coin
110 coin.center_x = random.randrange(SCREEN_WIDTH)
111 coin.center_y = random.randrange(-SCREEN_HEIGHT, 0)
112
113 # Add the coin to the lists
114 self.coin_list.append(coin)
115
116 def setup(self):
117 """ Set up the game and initialize the variables. """
118
119 self.score = 0
120 self.level = 1
121
122 # Sprite lists
123 self.player_list = arcade.SpriteList()
124 self.coin_list = arcade.SpriteList()
125
126 # Set up the player
127 self.player_sprite = arcade.Sprite(":resources:images/animated_characters/female_person/femalePerson_idle.png",
128 scale=SPRITE_SCALING)
129 self.player_sprite.center_x = 50
130 self.player_sprite.center_y = 50
131 self.player_list.append(self.player_sprite)
132
133 self.level_1()
134
135 def on_draw(self):
136 """
137 Render the screen.
138 """
139
140 # This command has to happen before we start drawing
141 self.clear()
142
143 # Draw all the sprites.
144 self.player_sprite.draw()
145 self.coin_list.draw()
146
147 # Put the text on the screen.
148 output = f"Score: {self.score}"
149 arcade.draw_text(output, 10, 20, arcade.color.WHITE, 15)
150
151 output = f"Level: {self.level}"
152 arcade.draw_text(output, 10, 35, arcade.color.WHITE, 15)
153
154 def on_mouse_motion(self, x, y, dx, dy):
155 """
156 Called whenever the mouse moves.
157 """
158 self.player_sprite.center_x = x
159 self.player_sprite.center_y = y
160
161 def on_update(self, delta_time):
162 """ Movement and game logic """
163
164 # Call update on all sprites (The sprites don't do much in this
165 # example though.)
166 self.coin_list.update()
167
168 # Generate a list of all sprites that collided with the player.
169 hit_list = arcade.check_for_collision_with_list(self.player_sprite, self.coin_list)
170
171 # Loop through each colliding sprite, remove it, and add to the score.
172 for coin in hit_list:
173 coin.remove_from_sprite_lists()
174 self.score += 1
175
176 # See if we should go to level 2
177 if len(self.coin_list) == 0 and self.level == 1:
178 self.level += 1
179 self.level_2()
180 # See if we should go to level 3
181 elif len(self.coin_list) == 0 and self.level == 2:
182 self.level += 1
183 self.level_3()
184
185
186def main():
187 """ Main function """
188 window = MyGame(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
189 window.setup()
190 arcade.run()
191
192
193if __name__ == "__main__":
194 main()