Collect Coins that are Bouncing#
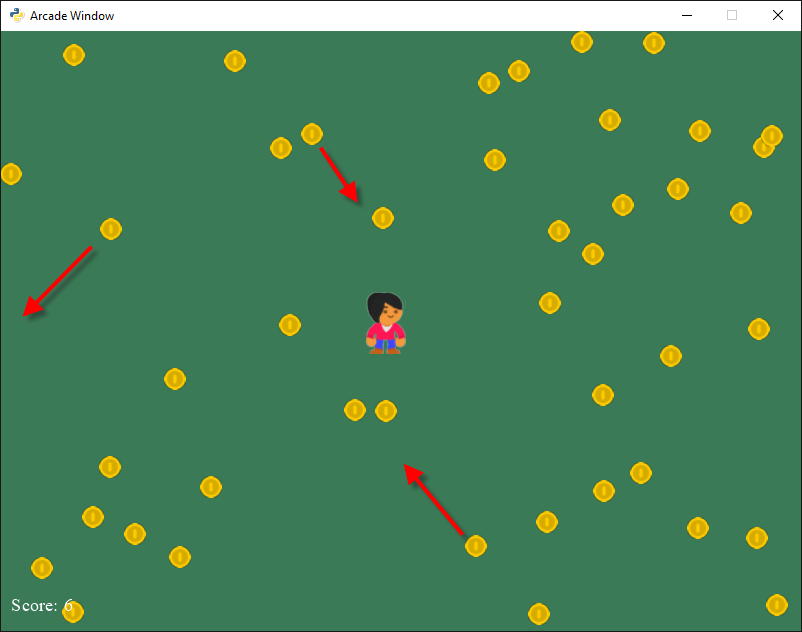
sprite_collect_coins_move_bouncing.py#
1"""
2Sprite Collect Moving and Bouncing Coins
3
4Simple program to show basic sprite usage.
5
6Artwork from https://kenney.nl
7
8If Python and Arcade are installed, this example can be run from the command line with:
9python -m arcade.examples.sprite_collect_coins_move_bouncing
10"""
11
12import random
13import arcade
14
15# --- Constants ---
16SPRITE_SCALING_PLAYER = 0.5
17SPRITE_SCALING_COIN = 0.2
18COIN_COUNT = 50
19
20SCREEN_WIDTH = 800
21SCREEN_HEIGHT = 600
22SCREEN_TITLE = "Sprite Collect Moving and Bouncing Coins Example"
23
24
25class Coin(arcade.Sprite):
26
27 def __init__(self, filename, scale):
28
29 super().__init__(filename, scale=scale)
30
31 self.change_x = 0
32 self.change_y = 0
33
34 def update(self):
35
36 # Move the coin
37 self.center_x += self.change_x
38 self.center_y += self.change_y
39
40 # If we are out-of-bounds, then 'bounce'
41 if self.left < 0:
42 self.change_x *= -1
43
44 if self.right > SCREEN_WIDTH:
45 self.change_x *= -1
46
47 if self.bottom < 0:
48 self.change_y *= -1
49
50 if self.top > SCREEN_HEIGHT:
51 self.change_y *= -1
52
53
54class MyGame(arcade.Window):
55 """ Our custom Window Class"""
56
57 def __init__(self):
58 """ Initializer """
59 # Call the parent class initializer
60 super().__init__(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_TITLE)
61
62 # Variables that will hold sprite lists
63 self.all_sprites_list = None
64 self.coin_list = None
65
66 # Set up the player info
67 self.player_sprite = None
68 self.score = 0
69
70 # Don't show the mouse cursor
71 self.set_mouse_visible(False)
72
73 self.background_color = arcade.color.AMAZON
74
75 def setup(self):
76 """ Set up the game and initialize the variables. """
77
78 # Sprite lists
79 self.all_sprites_list = arcade.SpriteList()
80 self.coin_list = arcade.SpriteList()
81
82 # Score
83 self.score = 0
84
85 # Set up the player
86 # Character image from kenney.nl
87 self.player_sprite = arcade.Sprite(
88 ":resources:images/animated_characters/female_person/femalePerson_idle.png",
89 scale=SPRITE_SCALING_PLAYER,
90 )
91 self.player_sprite.center_x = 50
92 self.player_sprite.center_y = 50
93 self.all_sprites_list.append(self.player_sprite)
94
95 # Create the coins
96 for i in range(50):
97
98 # Create the coin instance
99 # Coin image from kenney.nl
100 coin = Coin(":resources:images/items/coinGold.png", scale=SPRITE_SCALING_COIN)
101
102 # Position the coin
103 coin.center_x = random.randrange(SCREEN_WIDTH)
104 coin.center_y = random.randrange(SCREEN_HEIGHT)
105 coin.change_x = random.randrange(-3, 4)
106 coin.change_y = random.randrange(-3, 4)
107
108 # Add the coin to the lists
109 self.all_sprites_list.append(coin)
110 self.coin_list.append(coin)
111
112 def on_draw(self):
113 """ Draw everything """
114 self.clear()
115 self.all_sprites_list.draw()
116
117 # Put the text on the screen.
118 output = f"Score: {self.score}"
119 arcade.draw_text(output, 10, 20, arcade.color.WHITE, 14)
120
121 def on_mouse_motion(self, x, y, dx, dy):
122 """ Handle Mouse Motion """
123
124 # Move the center of the player sprite to match the mouse x, y
125 self.player_sprite.center_x = x
126 self.player_sprite.center_y = y
127
128 def on_update(self, delta_time):
129 """ Movement and game logic """
130
131 # Call update on all sprites (The sprites don't do much in this
132 # example though.)
133 self.all_sprites_list.update()
134
135 # Generate a list of all sprites that collided with the player.
136 hit_list = arcade.check_for_collision_with_list(self.player_sprite,
137 self.coin_list)
138
139 # Loop through each colliding sprite, remove it, and add to the score.
140 for coin in hit_list:
141 coin.remove_from_sprite_lists()
142 self.score += 1
143
144
145def main():
146 window = MyGame()
147 window.setup()
148 arcade.run()
149
150
151if __name__ == "__main__":
152 main()